mickel2000
SEO Keyword Architect
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
400 XP
Getting Started
I've used CDN for Bootstrap, Angular JS and Chart JS so you need internet connection for them to work.
Creating our Database
First, we're gonna create our MySQL Database where we get our data.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as angular.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
index.html
This is our index which contains our add form to update our chart and the chart itself.
app.js
This contains our angular js scripts.
fetchfruit.php
This is our PHP api that fetches data for our add form.
purchase.php
This is our PHP api/code that adds data into our database.
fetchsales.php
Lastly, this is our PHP api that fetches data for our chart.
That ends this tutorial. Happy Coding :)
Download
I've used CDN for Bootstrap, Angular JS and Chart JS so you need internet connection for them to work.
Creating our Database
First, we're gonna create our MySQL Database where we get our data.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as angular.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
- CREATE
TABLE
`fruits`
(
- `fruitid`
int
(
11
)
NOT
NULL
AUTO_INCREMENT
,
- `fruitname`
varchar
(
30
)
NOT
NULL
,
- PRIMARY KEY
(
`fruitid`
)
- )
ENGINE
=
InnoDB
DEFAULT
CHARSET
=
latin1;
- CREATE
TABLE
`sales`
(
- `saleid`
int
(
11
)
NOT
NULL
AUTO_INCREMENT
,
- `fruitid`
int
(
11
)
NOT
NULL
,
- `amount`
double
NOT
NULL
,
- PRIMARY KEY
(
`saleid`
)
- )
ENGINE
=
InnoDB
DEFAULT
CHARSET
=
latin1;
- INSERT
INTO
`fruits`
(
`fruitid`
,
`fruitname`
)
VALUES
- (
1
,
'Apple'
)
,
- (
2
,
'Orange'
)
,
- (
3
,
'Strawberry'
)
,
- (
4
,
'Mango'
)
;
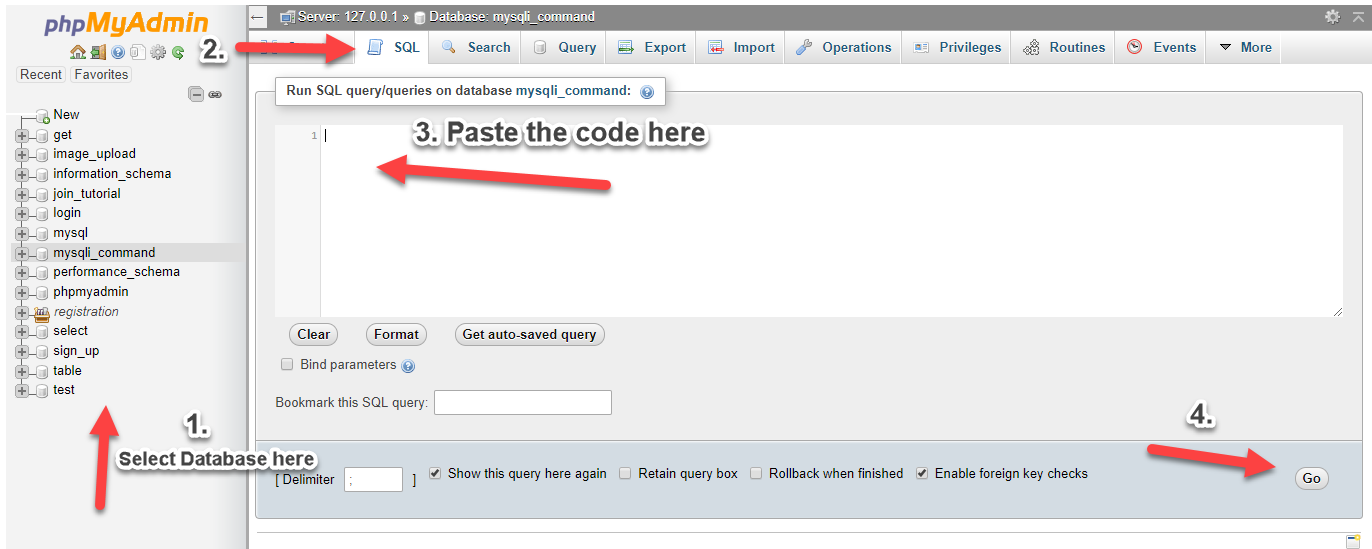
index.html
This is our index which contains our add form to update our chart and the chart itself.
- <!DOCTYPE html>
- <html
ng-app=
"app"
>
- <head
>
- <title
>
Pie/Doughnut Chart using ChartJS, AngularJS and PHP/MySQLi</
title
>
- <meta
charset
=
"utf-8"
>
- <link
href
=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"
rel
=
"stylesheet"
>
- <script
src
=
"http://ajax.googleapis.com/ajax/libs/angularjs/1.5.7/angular.min.js"
></
script
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.7.1/Chart.bundle.min.js"
></
script
>
- <style
type
=
"text/css"
>
- canvas{
- margin:auto;
- }
- .alert{
- margin-top:20px;
- }
- </
style
>
- </
head
>
- <body
ng-controller=
"myCtrl"
>
- <div
class
=
"container"
>
- <div
class
=
"row"
>
- <div
class
=
"col-sm-3 col-md-offset-1"
ng-init=
"fetchfruit()"
>
- <h3
class
=
"page-header text-center"
>
Add Purchase</
h3
>
- <div
class
=
"form-group"
>
- <label
>
Select Fruit:</
label
>
- <select
ng-model=
"buy.fruitid"
class
=
"form-control"
>
- <option
ng-repeat=
"fruit in fruits"
value
=
"{{fruit.fruitid}}"
>
{{fruit.fruitname}}</
option
>
- </
select
>
- </
div
>
- <div
class
=
"form-group"
>
- <label
>
Amount:</
label
>
- <input
type
=
"text"
class
=
"form-control"
ng-model=
"buy.amount"
>
- </
div
>
- <button
type
=
"button"
ng-click=
"purchase()"
class
=
"btn btn-primary"
>
Buy</
button
>
- <div
class
=
"alert alert-success text-center"
ng-show=
"success"
>
- <button
type
=
"button"
class
=
"close"
aria-hidden=
"true"
ng-click=
"clear()"
>
×
</
button
>
- {{ message }}
- </
div
>
- <div
class
=
"alert alert-danger text-center"
ng-show=
"error"
>
- <button
type
=
"button"
class
=
"close"
aria-hidden=
"true"
ng-click=
"clear()"
>
×
</
button
>
- {{ message }}
- </
div
>
- </
div
>
- <div
class
=
"col-sm-7"
ng-init=
"fetchsales()"
>
- <h3
class
=
"page-header text-center"
>
Sales Chart</
h3
>
- <canvas id
=
"dvCanvas"
height
=
"400"
width
=
"400"
></
canvas>
- </
div
>
- </
div
>
- </
div
>
- <script
src
=
"app.js"
></
script
>
- </
body
>
- </
html
>
app.js
This contains our angular js scripts.
- var
app =
angular.module
(
'app'
,
[
]
)
;
- app.controller
(
'myCtrl'
,
function
(
$scope,
$http)
{
- $scope.error
=
false
;
- $scope.success
=
false
;
- $scope.fetchfruit
=
function
(
)
{
- $http.get
(
'fetchfruit.php'
)
.success
(
function
(
data)
{
- $scope.fruits
=
data;
- }
)
;
- }
- $scope.purchase
=
function
(
)
{
- $http.post
(
'purchase.php'
,
$scope.buy
)
- .success
(
function
(
data)
{
- if
(
data.error
)
{
- $scope.error
=
true
;
- $scope.success
=
false
;
- $scope.message
=
data.message
;
- }
- else
{
- $scope.success
=
true
;
- $scope.error
=
false
;
- $scope.message
=
data.message
;
- $scope.fetchsales
(
)
;
- $scope.buy
=
''
;
- }
- }
)
;
- }
- //this fetches the data for our table
- $scope.fetchsales
=
function
(
)
{
- $http.get
(
'fetchsales.php'
)
.success
(
function
(
data)
{
- var
ctx =
document.getElementById
(
"dvCanvas"
)
.getContext
(
'2d'
)
;
- var
myChart =
new
Chart(
ctx,
{
- type:
'pie'
,
// change the value of pie to doughtnut for doughnut chart
- data:
{
- datasets:
[
{
- data:
data.total
,
- backgroundColor:
[
'blue'
,
'green'
,
'red'
,
'yellow'
]
- }
]
,
- labels:
data.fruitname
- }
,
- options:
{
- responsive:
false
- }
- }
)
;
- }
)
;
- }
- $scope.clear
=
function
(
)
{
- $scope.error
=
false
;
- $scope.success
=
false
;
- }
- }
)
;
fetchfruit.php
This is our PHP api that fetches data for our add form.
- <?php
- $conn
=
new
mysqli(
"localhost"
,
"root"
,
""
,
"angular"
)
;
- $out
=
array
(
)
;
- $sql
=
"SELECT * FROM fruits"
;
- $query
=
$conn
->
query
(
$sql
)
;
- while
(
$row
=
$query
->
fetch_array
(
)
)
{
- $out
[
]
=
$row
;
- }
- echo
json_encode
(
$out
)
;
- ?>
purchase.php
This is our PHP api/code that adds data into our database.
- <?php
- $conn
=
new
mysqli(
"localhost"
,
"root"
,
""
,
"angular"
)
;
- $out
=
array
(
'error'
=>
false
)
;
- $data
=
json_decode
(
file_get_contents
(
"php://input"
)
)
;
- $fruitid
=
$data
->
fruitid
;
- $amount
=
$data
->
amount
;
- $sql
=
"INSERT INTO sales (fruitid, amount) VALUES ('$fruitid
', '$amount
')"
;
- $query
=
$conn
->
query
(
$sql
)
;
- if
(
$query
)
{
- $out
[
'message'
]
=
"Purchase added successfully"
;
- }
- else
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
"Cannot add purchase"
;
- }
- echo
json_encode
(
$out
)
;
- ?>
fetchsales.php
Lastly, this is our PHP api that fetches data for our chart.
- <?php
- $conn
=
new
mysqli(
"localhost"
,
"root"
,
""
,
"angular"
)
;
- $out
=
array
(
)
;
- $sql
=
"SELECT *, sum(amount) AS total FROM sales LEFT JOIN fruits ON fruits.fruitid=sales.fruitid GROUP BY sales.fruitid"
;
- $query
=
$conn
->
query
(
$sql
)
;
- while
(
$row
=
$query
->
fetch_array
(
)
)
{
- $out
[
'total'
]
[
]
=
$row
[
'total'
]
;
- $out
[
'fruitname'
]
[
]
=
$row
[
'fruitname'
]
;
- }
- echo
json_encode
(
$out
)
;
- ?>
That ends this tutorial. Happy Coding :)
Download
You must upgrade your account or reply in the thread to view the hidden content.