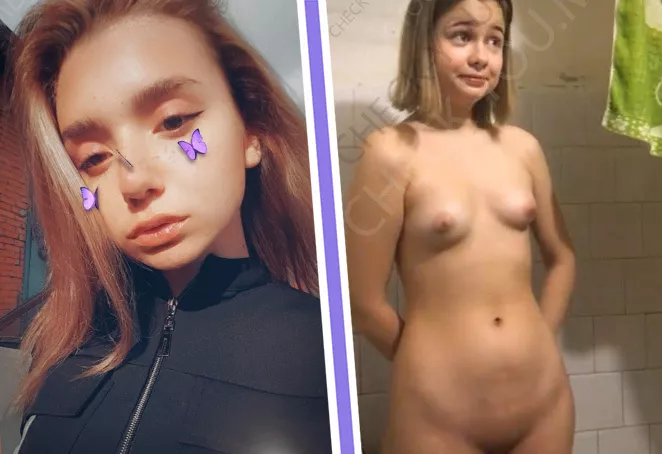
Leaked Huge mega Archive:
VIP & SUPREME Backup Links:
Ejvazova Marija, fansly, snapwins, leaked images, adult entertainers, onlyfans, , subscription-based service, premium archive, leaked videos, statewins, leaked, exclusive content, content creator leaks, adult directory, adult content