typicalclout
Smart Infrastructure Designer
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
In this tutorial, you will learn a simple way to print the specific element in your web applications page using JavaScript and jQuery. This technique is useful for your future project for printing reports that have already a display. With this, it helps you to prevent redundant coding just for creating a page for printing. Using this printing technique developer can also modify or customize the element before printing and without changing the original display.
Library Needed:
Method Use:
Printing Code
Below is the example js script for printing the specific element on the window page. The script also includes all the styles and script loaded on the page to maintaint the design of the element to print.
I will be providing below a simple HTML and JavaScript Source Code file for a simple web application that contains a feature that meets our goal for this tutorial. The source code only prints the specific element but maintains the element design.
Main Interface
The following script is the HTML file naming index.html. This file contains the HTML Scripts of the elements that are shown when browsing the page.
Page Display Snapshot
Main Script
The following script is a JavaScript naming script.js. This file is included and loaded in the index.html file. It contains the JavaScript codes for printing the element file.
Print View Snapshot
That's it! You can now test the source code on your end by browsing the index.html file in your preferred browser such as Chrome Browser. If you have encountered an error on your end, please review the changes you made to the scripts and differentiate them from the source code I provided above. You can also download the zip file of the source code I created for this tutorial. The download button is located below this article.
DEMO VIDEO
That's the end of this tutorial! I hope this JavaScript Tutorial for Printing the HTML Element will help you with what you are looking for and you'll find this tutorial useful for your future web application projects. Explore more on this website for more Tutorials and Free Source Codes..
Enjoy Coding:)
Download
Library Needed:
Method Use:
- JS clone()
- JS window.open()
- JS setTimeout()
- DOM Manipulation
Printing Code
Below is the example js script for printing the specific element on the window page. The script also includes all the styles and script loaded on the page to maintaint the design of the element to print.
- function
print_el(
)
{
- //HTML head tag that contains the loaded stylesheets and scritps
- var
header =
$(
'head'
)
.clone
(
)
- //Cloning the Element to Print
- var
el =
$(
'#printout'
)
.clone
(
)
- //Opening a new window
- var
nw =
window.open
(
''
,
'_blank'
,
"width="
+
(
$(
window)
.width
(
)
*
.8)
+
", left="
+
(
$(
window)
.width
(
)
*
.1)
+
",height="
+
(
$(
window)
.height
(
)
*
.8)
+
", top="
+
(
$(
window)
.height
(
)
*
.1)
)
- //Manipulating the New Window head tag
- nw.document
.querySelector
(
'head'
)
.innerHTML
=
header.html
(
)
- //Manipulating the New Window content
- nw.document
.querySelector
(
'body'
)
.innerHTML
=
el[
0
]
.outerHTML
- nw.document
.close
(
)
- setTimeout(
(
)
=>
{
- //Printing the New Window
- nw.print
(
)
- setTimeout(
(
)
=>
{
- nw.close
(
)
- }
,
300
)
- }
,
300
)
- }
I will be providing below a simple HTML and JavaScript Source Code file for a simple web application that contains a feature that meets our goal for this tutorial. The source code only prints the specific element but maintains the element design.
Main Interface
The following script is the HTML file naming index.html. This file contains the HTML Scripts of the elements that are shown when browsing the page.
- <!DOCTYPE html>
- <html
lang
=
"en"
>
- <head
>
- <meta
charset
=
"UTF-8"
>
- <meta
http-equiv
=
"X-UA-Compatible"
content
=
"IE=edge"
>
- <meta
name
=
"viewport"
content
=
"width=device-width, initial-scale=1.0"
>
- <title
>
JS Custom Print</
title
>
- <!-- Styles -->
- <link
rel
=
"stylesheet"
href
=
"https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css"
integrity=
"sha512-KfkfwYDsLkIlwQp6LFnl8zNdLGxu9YAA1QvwINks4PhcElQSvqcyVLLD9aMhXd13uQjoXtEKNosOWaZqXgel0g=="
crossorigin=
"anonymous"
referrerpolicy=
"no-referrer"
- /
>
- <link
rel
=
"stylesheet"
href
=
"https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css"
integrity=
"sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3"
crossorigin=
"anonymous"
>
- <!--end of Styles -->
- <!-- Scritps -->
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"
integrity=
"sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ=="
crossorigin=
"anonymous"
referrerpolicy=
"no-referrer"
></
script
>
- <script
src
=
"https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"
integrity=
"sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p"
crossorigin=
"anonymous"
></
script
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/js/all.min.js"
integrity=
"sha512-6PM0qYu5KExuNcKt5bURAoT6KCThUmHRewN3zUFNaoI6Di7XJPTMoT6K0nsagZKk2OB4L7E3q1uQKHNHd4stIQ=="
crossorigin=
"anonymous"
referrerpolicy=
"no-referrer"
></
script
>
- <script
src
=
"./script.js"
></
script
>
- <!-- end of Scritps -->
- </
head
>
- <body
class
=
"bg-gradient-dark"
>
- <div
class
=
"content py-4"
>
- <div
class
=
"container"
>
- <div
class
=
"card rounded-0"
>
- <div
class
=
"card-header"
>
- <div
class
=
"d-flex w-100 align-items-center"
>
- <div
class
=
"col-auto flex-shrink-1 flex-grow-1"
>
- <div
class
=
"card-title"
><b
>
Printing Page Element using JavaScrip</
b
></
div
>
- </
div
>
- <div
class
=
"col-auto"
>
- <button
class
=
"btn btn-success bg-gradient bg-success btn-sm rounded-0"
type
=
"button"
id
=
"print"
><i
class
=
"fa fa-print"
></
i
>
Print Data</
button
>
- </
div
>
- </
div
>
- </
div
>
- <div
class
=
"card-body"
>
- <div
class
=
"container-fluid"
>
- <h5
class
=
"fw-bolder"
>
Data to Print:</
h5
>
- <div
class
=
"border rouded-0 border-dark p-2"
>
- <div
id
=
"printout"
>
- <div
class
=
"lh-1"
>
- <h1
class
=
"text-center"
>
Lorem Ipsum</
h1
>
- <h4
class
=
"text-center mb-0"
>
"Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit..."</
h4
>
- <h5
class
=
"text-center fw-lighter mb-0"
>
"There is no one who loves pain itself, who seeks after it and wants to have it, simply because it is pain..."</
h5
>
- </
div
>
- <hr
>
- <p
>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed ac nisl nibh. Pellentesque sit amet orci lacinia, facilisis tortor in, ultrices enim. Maecenas elementum metus vitae lacus sodales viverra. Cras in ultrices orci,
- vel sollicitudin arcu. Aliquam erat volutpat. Praesent in nisi magna. In vel urna et turpis lobortis hendrerit in sed est. Aliquam eu enim felis. Vivamus eget accumsan ante. Pellentesque ut laoreet magna. Maecenas quis
- magna ac est finibus pharetra. Curabitur accumsan faucibus nunc, blandit congue tellus consectetur vitae. Proin auctor risus vel orci ornare, sit amet vestibulum nunc scelerisque. Suspendisse consectetur mi sit amet
- nisl sodales, in faucibus mi placerat. In maximus efficitur metus quis venenatis.</
p
>
- <p
>
Duis placerat id dui in vestibulum. Vestibulum ante ipsum primis in faucibus orci luctus et ultrices posuere cubilia curae; Suspendisse sagittis, sapien sit amet congue blandit, leo nunc convallis mauris, ac lacinia nunc
- sem quis nulla. Nullam viverra, dui eu dapibus pellentesque, dolor diam luctus risus, a posuere diam nisi ac ligula. Sed at augue ut mauris lacinia pellentesque non sit amet ipsum. Donec sollicitudin faucibus facilisis.
- Sed viverra imperdiet arcu eget lacinia. Pellentesque ultricies rhoncus quam non feugiat. Etiam commodo lorem ac risus aliquam aliquam. Pellentesque eget nibh a nunc dapibus consectetur. Aliquam sapien turpis, molestie
- vel nunc vitae, placerat sollicitudin enim. Vivamus et tellus rhoncus diam rhoncus tristique et et dui. Nunc mattis, purus et mollis luctus, urna massa placerat mi, vel facilisis magna ligula ut felis. Orci varius natoque
- penatibus et magnis dis parturient montes, nascetur ridiculus mus.</
p
>
- <h3
><b
>
Table</
b
></
h3
>
- <hr
>
- <table
class
=
"table table-stripped table-bordered"
>
- <thead
>
- <tr
class
=
"bg-gradient bg-danger bg-opacity-25"
>
- <th
class
=
"py-1 text-center"
>
#</
th
>
- <th
class
=
"py-1 text-center"
>
Name</
th
>
- <th
class
=
"py-1 text-center"
>
Contact</
th
>
- <th
class
=
"py-1 text-center"
>
Address</
th
>
- </
tr
>
- </
thead
>
- <tbody
>
- <tr
>
- <th
class
=
"py-1 text-center"
>
1</
th
>
- <th
class
=
"py-1"
>
Mark Cooper</
th
>
- <th
class
=
"py-1"
>
09123456789</
th
>
- <th
class
=
"py-1"
>
Sample Address #101</
th
>
- </
tr
>
- <tr
>
- <th
class
=
"py-1 text-center"
>
2</
th
>
- <th
class
=
"py-1"
>
Samantha Jane Miller</
th
>
- <th
class
=
"py-1"
>
09123654789</
th
>
- <th
class
=
"py-1"
>
Sample Address #102</
th
>
- </
tr
>
- <tr
>
- <th
class
=
"py-1 text-center"
>
3</
th
>
- <th
class
=
"py-1"
>
John Doe</
th
>
- <th
class
=
"py-1"
>
09456789123</
th
>
- <th
class
=
"py-1"
>
Sample Address #102</
th
>
- </
tr
>
- </
tbody
>
- </
table
>
- </
div
>
- </
div
>
- </
div
>
- </
div
>
- </
div
>
- </
div
>
- </
div
>
- </
body
>
- </
html
>
Page Display Snapshot
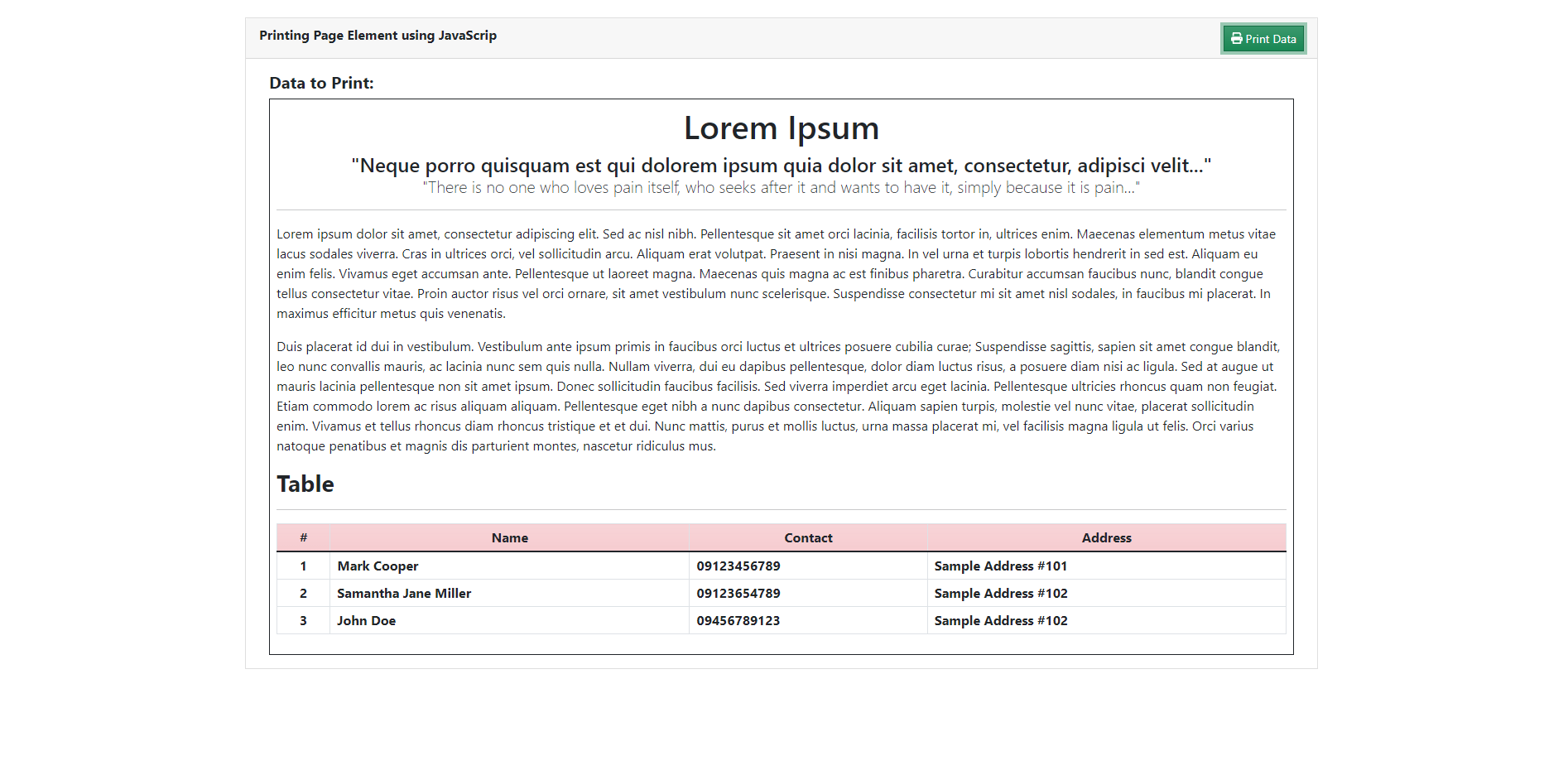
Main Script
The following script is a JavaScript naming script.js. This file is included and loaded in the index.html file. It contains the JavaScript codes for printing the element file.
- $(
function
(
)
{
- $(
'#print'
)
.click
(
function
(
)
{
- var
header =
$(
'head'
)
.clone
(
)
- var
el =
$(
'#printout'
)
.clone
(
)
- var
nw =
window.open
(
''
,
'_blank'
,
"width="
+
(
$(
window)
.width
(
)
*
.8)
+
", left="
+
(
$(
window)
.width
(
)
*
.1)
+
",height="
+
(
$(
window)
.height
(
)
*
.8)
+
", top="
+
(
$(
window)
.height
(
)
*
.1)
)
- nw.document
.querySelector
(
'head'
)
.innerHTML
=
header.html
(
)
- nw.document
.querySelector
(
'body'
)
.innerHTML
=
el[
0
]
.outerHTML
- nw.document
.close
(
)
- setTimeout(
(
)
=>
{
- nw.print
(
)
- setTimeout(
(
)
=>
{
- nw.close
(
)
- }
,
300
)
- }
,
300
)
- }
)
- }
)
Print View Snapshot
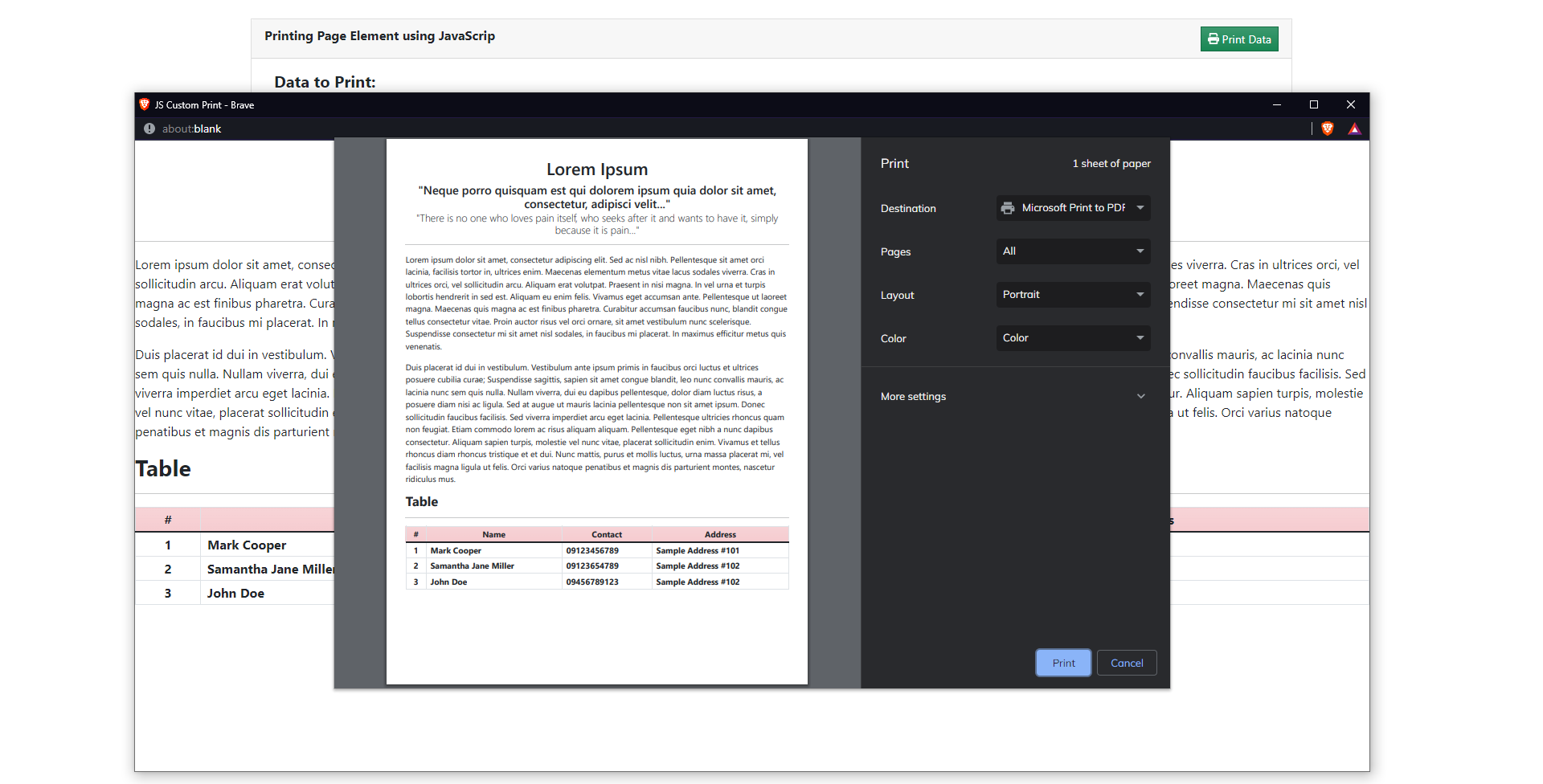
That's it! You can now test the source code on your end by browsing the index.html file in your preferred browser such as Chrome Browser. If you have encountered an error on your end, please review the changes you made to the scripts and differentiate them from the source code I provided above. You can also download the zip file of the source code I created for this tutorial. The download button is located below this article.
DEMO VIDEO
That's the end of this tutorial! I hope this JavaScript Tutorial for Printing the HTML Element will help you with what you are looking for and you'll find this tutorial useful for your future web application projects. Explore more on this website for more Tutorials and Free Source Codes..
Enjoy Coding:)
Download
You must upgrade your account or reply in the thread to view the hidden content.