SaltLad
Shonen Battle Strategist
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
If you want to display the latest transaction on the top of the ListView, this tutorial is just right for you. So, in this tutorial, I will teach you how to sort records in a ListView in descending order using C#. This method has the ability to retrieve the data in the database and display it into the ListView that will automatically sort the latest date in which the transaction had been made. In this way, you don’t have to scroll down the records in the ListView because it will place the latest record on the top of the ListView when the transaction is made.
Creating a Database
Create a database in the “PHPMyAdmin” and named it “dbtransaction”.
After that, execute the following query to create a table in the database that you have created.
Insert the data in the table.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.
Step 2
Add a ListView in the form. After that do the form just like shown below.
Step 3
Add MySQL.Data.dll as references.
Step 4
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL
libraries.
Step 5
Create a connection between c# and MySQL database and declare all the classes that are needed.
Step 6
Create a method for retrieving data in the database and display it in the ListView in descending order.
Step 7
Write the following codes to display the data in the ListView in the first load of the form.
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Download
Creating a Database
Create a database in the “PHPMyAdmin” and named it “dbtransaction”.
After that, execute the following query to create a table in the database that you have created.
- CEATE TABLE
`tbltransaction`
(
- `TRANSID`
int
(
11
)
NOT
NULL
,
- `ORNO`
int
(
30
)
NOT
NULL
,
- `TRANSDATE`
date
NOT
NULL
,
- `AMOUNTSALE`
double
NOT
NULL
,
- `CASHIER`
varchar
(
30
)
NOT
NULL
- )
ENGINE
=
InnoDB
DEFAULT
CHARSET
=
latin1;
Insert the data in the table.
- INSERT
INTO
`tbltransaction`
(
`TRANSID`
,
`ORNO`
,
`TRANSDATE`
,
`AMOUNTSALE`
,
`CASHIER`
)
VALUES
- (
1
,
70004
,
'2019-02-21'
,
385
,
'Janno Palacios'
)
,
- (
2
,
70005
,
'2019-02-21'
,
385
,
'Janno Palacios'
)
,
- (
3
,
70002
,
'2019-02-17'
,
385
,
'Janno Palacios'
)
,
- (
4
,
70001
,
'2019-02-18'
,
385
,
'Janno Palacios'
)
,
- (
5
,
70006
,
'2019-02-19'
,
69
,
'Janno Palacios'
)
,
- (
6
,
70007
,
'2019-02-21'
,
69
,
'Janno Palacios'
)
,
- (
7
,
70003
,
'2019-02-07'
,
138
,
'Janno Palacios'
)
;
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application in c#.
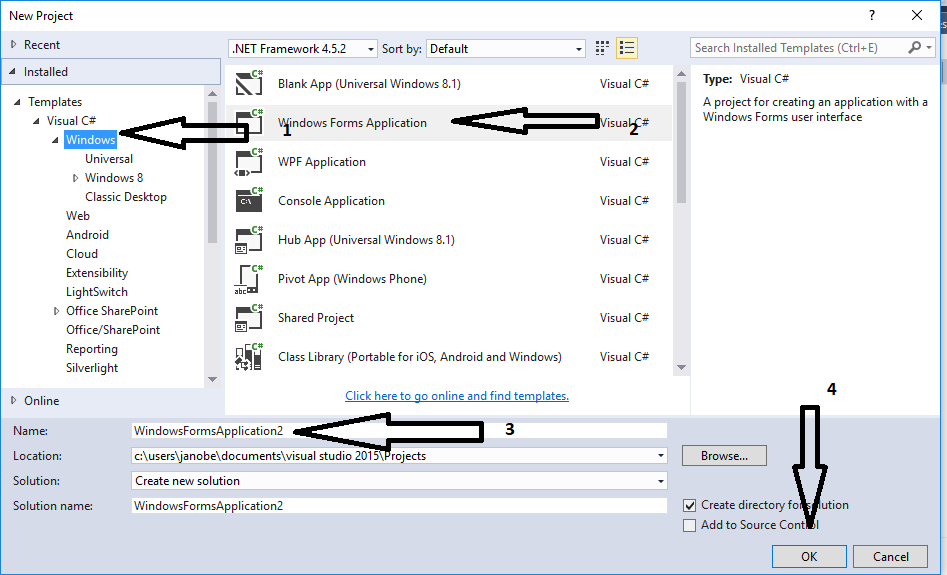
Step 2
Add a ListView in the form. After that do the form just like shown below.
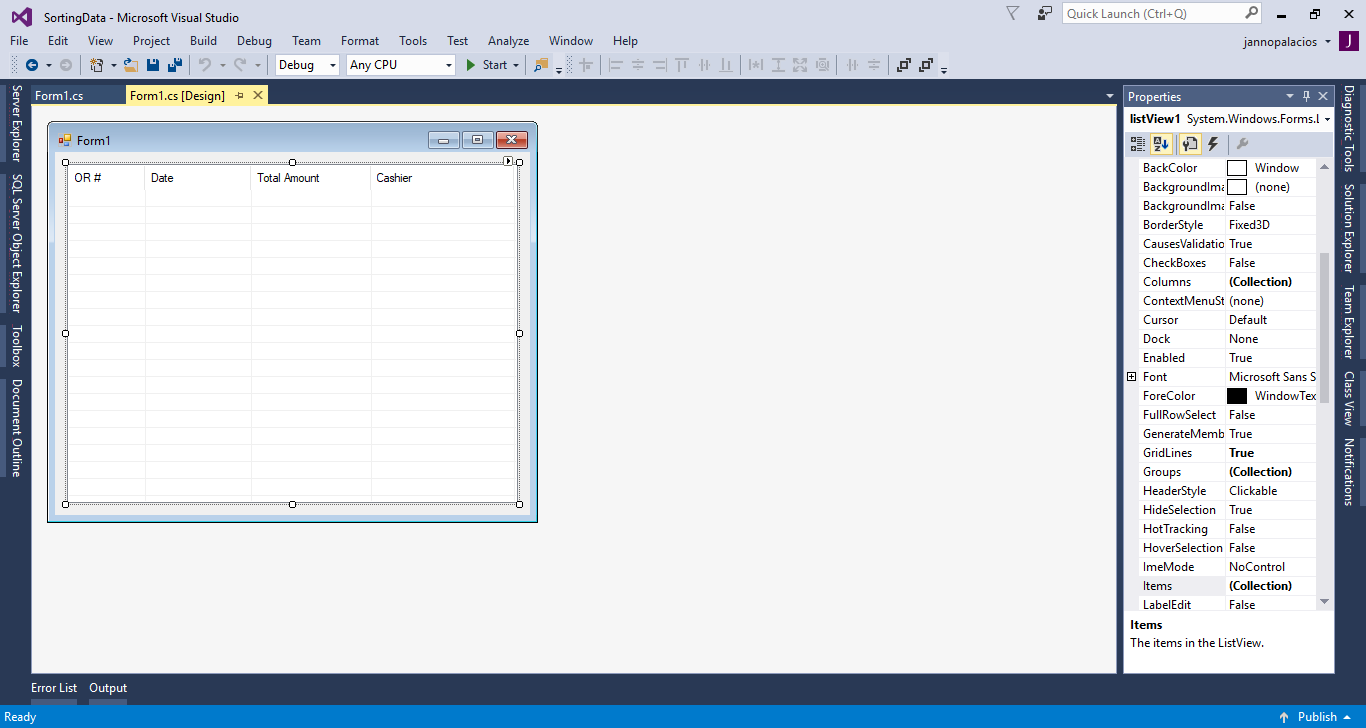
Step 3
Add MySQL.Data.dll as references.
Step 4
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL
libraries.
- using
MySql.Data.MySqlClient
;
Step 5
Create a connection between c# and MySQL database and declare all the classes that are needed.
- MySqlConnection con =
new
MySqlConnection(
"server=localhost;user id=root;password=;database=dbtransaction;sslMode=none"
)
;
- MySqlCommand cmd =
new
MySqlCommand(
)
;
- MySqlDataAdapter da =
new
MySqlDataAdapter(
)
;
- DataTable dt =
new
DataTable(
)
;
- string
sql;
Step 6
Create a method for retrieving data in the database and display it in the ListView in descending order.
- private
void
RetrieveDescending(
)
- {
- try
- {
- con.
Open
(
)
;
- cmd =
new
MySqlCommand(
)
;
- da =
new
MySqlDataAdapter(
)
;
- dt =
new
DataTable(
)
;
- sql =
"SELECT * FROM `tbltransaction` ORDER BY TRANSDATE DESC"
;
- cmd.
Connection
=
con;
- cmd.
CommandText
=
sql;
- da.
SelectCommand
=
cmd;
- da.
Fill
(
dt)
;
- listView1.
Items
.
Clear
(
)
;
- foreach
(
DataRow r in
dt.
Rows
)
- {
- ListViewItem list =
listView1.
Items
.
Add
(
r.
Field
<
int
>
(
1
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
DateTime>
(
2
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
Double
>
(
3
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
string
>
(
4
)
)
;
- }
- }
- catch
(
Exception ex)
- {
- MessageBox.
Show
(
ex.
Message
)
;
- }
- finally
- {
- con.
Close
(
)
;
- da.
Dispose
(
)
;
- }
- }
Step 7
Write the following codes to display the data in the ListView in the first load of the form.
- private
void
Form1_Load(
object
sender, EventArgs e)
- {
- RetrieveDescending(
)
;
- }
The complete source code is included. You can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Download
You must upgrade your account or reply in the thread to view the hidden content.