AccDispenser
API Endpoint Tester
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
300 XP
In this tutorial, I will teach you how to save data in the database using C#.net and SQL Server 2005 Express Edition. With this, you can access and save the data in the Server database with ease. This is the basic implementation for a newbie in programming who plans to build a system in the future.
break
Let's get started:
1. Create a database and name it "userdb".
2. Do this following query for creating a table in the database that you have created.
3. Open Microsoft Visual Studio 2008, create a new windows form application and do the form just like this.
4. Go to the Solution Explorer, click the “View Code” to fire the code editor.
5. Declare and initialize the classes that are needed.
Note: Add "using System.Data.SqlClient;" above the namespace to access sql server library.
6. Establish a connection between SQL Server and C#.net on the first load of the form.
7. Go back to the Design Views, Double click the "Save" button and create a method for saving the data in the SQL database.
Output:
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
break
Let's get started:
1. Create a database and name it "userdb".
2. Do this following query for creating a table in the database that you have created.
- /****** Object: Table [dbo].[tbluser] Script Date: 06/23/2016 08:36:33 ******/
- SET
ANSI_NULLS ON
- GO
- SET
QUOTED_IDENTIFIER ON
- GO
- CREATE
TABLE
[
dbo]
.
[
tbluser]
(
- [
ID]
[
INT
]
IDENTITY
(
1
,
1
)
NOT
NULL
,
- [
Name]
[
nvarchar]
(
50
)
NULL
,
- [
UNAME]
[
nvarchar]
(
50
)
NULL
,
- [
PASS]
[
nvarchar]
(
MAX
)
NULL
,
- [
UTYPE]
[
NCHAR
]
(
20
)
NULL
,
- CONSTRAINT
[
PK_tbluser]
PRIMARY
KEY
CLUSTERED
- (
- [
ID]
ASC
- )
WITH
(
PAD_INDEX =
OFF,
STATISTICS_NORECOMPUTE =
OFF,
IGNORE_DUP_KEY =
OFF,
ALLOW_ROW_LOCKS =
ON
,
ALLOW_PAGE_LOCKS =
ON
)
ON
[
PRIMARY
]
- )
ON
[
PRIMARY
]
3. Open Microsoft Visual Studio 2008, create a new windows form application and do the form just like this.
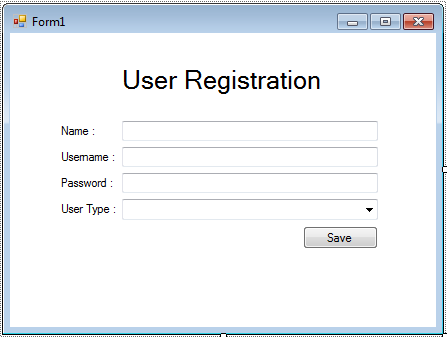
4. Go to the Solution Explorer, click the “View Code” to fire the code editor.
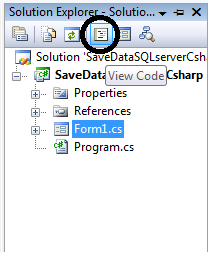
5. Declare and initialize the classes that are needed.
- //initialize all classes
- SqlConnection string_con =
new
SqlConnection(
)
;
- SqlCommand sql_command =
new
SqlCommand(
)
;
Note: Add "using System.Data.SqlClient;" above the namespace to access sql server library.
6. Establish a connection between SQL Server and C#.net on the first load of the form.
- private
void
Form1_Load(
object
sender, EventArgs e)
- {
- //bridge between sql server to c#
- string_con.
ConnectionString
=
"Data Source=.\\
SQLEXPRESS;Database=userdb;trusted_connection=true;"
;
- }
7. Go back to the Design Views, Double click the "Save" button and create a method for saving the data in the SQL database.
- private
void
btnSave_Click(
object
sender, EventArgs e)
- {
- try
- {
- //opening connection
- string_con .
Open
(
)
;
- //create an insert query;
- string
sql=
"INSERT INTO tbluser (NAME,UNAME,PASS,UTYPE) VALUES('"
+
txtName.
Text
+
"','"
+
txtUsername.
Text
+
"','"
+
txtPassword.
Text
+
"','"
+
cboType.
Text
+
"')"
;
- //initialize a new instance of sqlcommand
- sql_command =
new
SqlCommand(
)
;
- //set a connection used by this instance of sqlcommand
- sql_command .
Connection
=
string_con ;
- //set the sql statement to execute at the data source
- sql_command .
CommandText
=
sql;
- //execute the data.
- int
result =
sql_command.
ExecuteNonQuery
(
)
;
- //validate the result of the executed query.
- if
(
result >
0
)
- {
- MessageBox.
Show
(
"Data has been saved in the SQL database"
)
;
- }
- else
- {
- MessageBox.
Show
(
"SQL QUERY ERROR"
)
;
- }
- //closing connection
- string_con .
Close
(
)
;
- }
- catch
(
Exception ex)
//catch exeption
- {
- //displaying errors message.
- MessageBox.
Show
(
ex.
Message
)
;
- }
- }
Output:
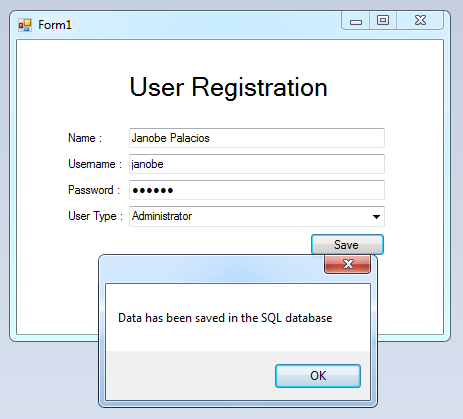
For all students who need a programmer for your thesis system or anyone who needs a source code in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT