Death10
AI-Driven OSINT Specialist
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
300 XP
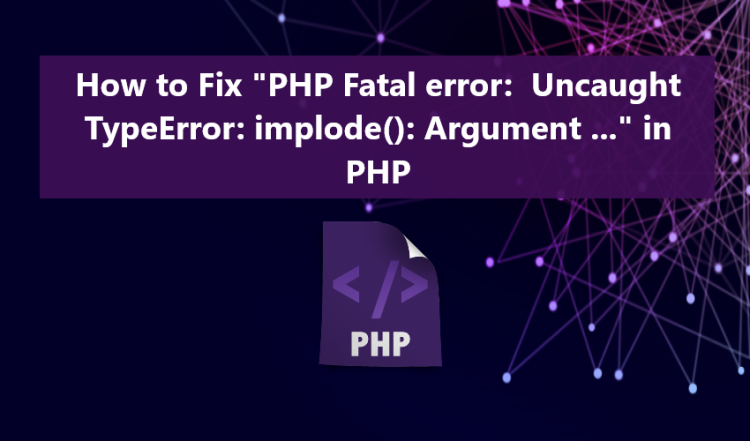
This article delves into understanding the causes of the PHP Error identified as "PHP Fatal error: Uncaught TypeError: implode(): Argument ...". We will also examine different solutions to counter this error encountered in PHP development. Within this article, I will present a PHP code sample that recreates the error scenario, along with code snippets that offer solutions.
What is PHP implode()
?
PHP provides a collection of valuable built-in functions, and one of these is the implode() function. The implode function is utilized to merge or join the values of an array into a single string. Furthermore, it enables the inclusion of a separator for individual values within the array. Using PHP implode() function, we can seamlessly combine the elements within an array into a unified string without encountering any difficulties.
Why does the "PHP Fatal error: Uncaught TypeError: implode(): Argument ..."
?
The "PHP Fatal error: Uncaught TypeError: implode(): Argument ..." PHP error is equivalent to the Warning: implode(): Invalid Arguments Passed error. This error emerges when trying to execute the implode function with inappropriate arguments. The implode function is designed solely for arrays; attempting to use other data types will trigger this error.
Here's a sample PHP snippet that simulates the error:
- <?php
- // Sample data
- $array
=
(
object)
[
"test"
,
"Hello World"
]
;
- // Execute and output implode data
- echo
implode
(
" | "
,
$array
)
;
- ?>
The implode() function has two arguments. The initial argument is a string utilized as a separator during the joining process, while the second argument is the array intended for joining.
Solutions
To fix the PHP Fatal error: Uncaught TypeError: implode(): Argument ..., it's crucial to adhere to the requisite argument types for the function. Presented here is a list of solutions to solve this error:
Verify that the first argument is a string and the second argument is an array
- <?php
- // Sample data
- $array
=
[
"test"
,
"Hello World"
]
;
- // Execute and output implode data
- echo
implode
(
" | "
,
$array
)
;
- ?>
If you passed an object as the second argument, convert it to an array
- <?php
- // Sample data
- $array
=
(
object)
[
"a"
=>
"test"
,
"b"
=>
"Hello World"
]
;
- // Execute and output implode data
- echo
implode
(
" | "
,
(
array
)
$array
)
;
- ?>
If you wish to join the keys of an object, you can employ the array_keys() function.
- <?php
- // Sample data
- $array
=
(
object)
[
"a"
=>
"test"
,
"b"
=>
"Hello World"
]
;
- // Execute and output implode data
- echo
implode
(
" | "
,
array_keys
(
(
array
)
$array
)
)
;
- ?>
Conclusion
In other words, the PHP Fatal error: Uncaught TypeError: implode(): Argument ... error emerges when we try to use the implode() function with an inappropriate argument type. The solution for this error entails ensuring that the argument types are correct: a string for the separator argument and an array to be joined. An additional approach to prevent this error is to include conditional checks before executing the implode function.
Explore more on this website for more Free Source Codes, Tutorials, and some Articles for fixing errors that you may encounter in your project development such as the following:
- Fixing the Notice: Undefined index
- Fixing the Fatal error: Uncaught TypeError: Illegal offset type
- Fixing the Warning: Array to string conversion