Drizzydrane
Meme Machine
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
This tutorial is a continuation of our last topic called “How to Create a Simple Record Navigation in C#”. But at this moment, we will modify our code and add more additional control such as First Record, Last Record and No of Record. And this look like as shown below.
To start building with this application, let’s open first our last project called “student_info”. Then add Buttons and Label. Next, arrange and Design it the same as shown above figure.
This time we will add functionality to our four buttons such as First, Previous, Next and Last. To start with, double click the “First record” button. Then add the following code:
Then for the “Previous Record” Button. Add the following code:
For the “Next Record” Button. Add the following code:
And lastly for “Last Record” Button. Here’s the following code:
At this time, you now test the application by pressing the “F5” or the Start button.
And here’s all the code used for this application.
Download
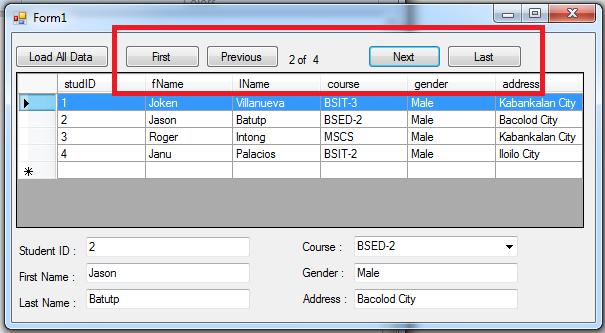
To start building with this application, let’s open first our last project called “student_info”. Then add Buttons and Label. Next, arrange and Design it the same as shown above figure.
This time we will add functionality to our four buttons such as First, Previous, Next and Last. To start with, double click the “First record” button. Then add the following code:
- private
void
btnfirst_Click(
object
sender, EventArgs e)
- {
- //check if the current record is not equal to zero
- if
(
curRecord !=
0
)
- //set the current record to zero
- curRecord =
0
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
Then for the “Previous Record” Button. Add the following code:
- private
void
btnprev_Click(
object
sender, EventArgs e)
- {
- //check if the current record is greater than zero
- if
(
curRecord >
0
)
- {
- //then decrement the current record by one
- curRecord =
curRecord -
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- }
For the “Next Record” Button. Add the following code:
- private
void
btnnext_Click(
object
sender, EventArgs e)
- {
- //check if the current record is not equal to the total Record minus by one
- if
(
curRecord !=
totRecord -
1
)
- {
- //increment the current record by one
- curRecord =
curRecord +
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- }
And lastly for “Last Record” Button. Here’s the following code:
- private
void
btnlast_Click(
object
sender, EventArgs e)
- {
- //check if the current Record is not Equal to the total record
- if
(
curRecord !=
totRecord)
- //set the current record equal to total record
- curRecord =
totRecord -
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
At this time, you now test the application by pressing the “F5” or the Start button.
And here’s all the code used for this application.
- using
System
;
- using
System.Collections.Generic
;
- using
System.ComponentModel
;
- using
System.Data
;
- using
System.Drawing
;
- using
System.Linq
;
- using
System.Text
;
- using
System.Windows.Forms
;
- using
System.Data.OleDb
;
- namespace
student_info
- {
- public
partial
class
Form1 :
Form
- {
- //declare new variable named dt as New Datatable
- DataTable dt =
new
DataTable(
)
;
- //this line of code used to connect to the server and locate the database (usermgt.mdb)
- static
string
connection =
"Provider=Microsoft.Jet.OLEDB.4.0;Data Source= "
+
Application.
StartupPath
+
"/studentdb.mdb"
;
- OleDbConnection conn =
new
OleDbConnection(
connection)
;
- int
curRecord =
0
;
- int
totRecord =
0
;
- public
Form1(
)
- {
- InitializeComponent(
)
;
- }
- private
void
btnload_Click(
object
sender, EventArgs e)
- {
- //create a new datatable
- dt =
new
DataTable(
)
;
- //create our SQL SELECT statement
- string
sql =
"Select * from tblstudent"
;
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- OleDbDataAdapter da =
new
OleDbDataAdapter(
sql, conn)
;
- //we fill the result to dt which declared above as datatable
- da.
Fill
(
dt)
;
- //set the curRecord to zero
- curRecord =
0
;
- //get the total number of record available in the database and stored to totRecord Variable
- totRecord =
dt.
Rows
.
Count
;
- //then we populate the datagridview by specifying the datasource equal to dt
- dataGridView1.
DataSource
=
dt;
- }
- private
void
dataGridView1_CellClick(
object
sender, DataGridViewCellEventArgs e)
- {
- //it checks if the row index of the cell is greater than or equal to zero
- if
(
e.
RowIndex
>=
0
)
- {
- //gets a collection that contains all the rows
- DataGridViewRow row =
this
.
dataGridView1
.
Rows
[
e.
RowIndex
]
;
- //populate the textbox from specific value of the coordinates of column and row.
- txtid.
Text
=
row.
Cells
[
0
]
.
Value
.
ToString
(
)
;
- txtfname.
Text
=
row.
Cells
[
1
]
.
Value
.
ToString
(
)
;
- txtlname.
Text
=
row.
Cells
[
2
]
.
Value
.
ToString
(
)
;
- comboBox1.
Text
=
row.
Cells
[
3
]
.
Value
.
ToString
(
)
;
- txtgender.
Text
=
row.
Cells
[
4
]
.
Value
.
ToString
(
)
;
- txtaddress.
Text
=
row.
Cells
[
5
]
.
Value
.
ToString
(
)
;
- }
- }
- private
void
Form1_Load(
object
sender, EventArgs e)
- {
- loadDatatoCombobox(
)
;
- }
- private
void
loadDatatoCombobox(
)
- {
- //create a new datatable
- DataTable table =
new
DataTable(
)
;
- //create our SQL SELECT statement
- string
sql =
"Select course from tblstudent"
;
- try
- {
- conn.
Open
(
)
;
- //then we execute the SQL statement against the Connection using OleDBDataAdapter
- OleDbDataAdapter da =
new
OleDbDataAdapter(
sql, conn)
;
- //we fill the result to dt which declared above as datatable
- da.
Fill
(
table)
;
- //we add new entry to our datatable manually
- //becuase Select Course is not Available in the Database
- table.
Rows
.
Add
(
"Select Course"
)
;
- //set the combobox datasource
- comboBox1.
DataSource
=
table;
- //choose the specific field to display
- comboBox1.
DisplayMember
=
"course"
;
- comboBox1.
ValueMember
=
"course"
;
- //set default selected value
- comboBox1.
SelectedValue
=
"Select Course"
;
- }
- catch
(
Exception ex)
- {
- //this will display some error message if something
- //went wrong to our code above during execution
- MessageBox.
Show
(
ex.
ToString
(
)
)
;
- }
- }
- private
void
Navigate(
)
- {
- txtid.
Text
=
dt.
Rows
[
curRecord]
[
0
]
.
ToString
(
)
;
- txtfname.
Text
=
dt.
Rows
[
curRecord]
[
1
]
.
ToString
(
)
;
- txtlname.
Text
=
dt.
Rows
[
curRecord]
[
2
]
.
ToString
(
)
;
- comboBox1.
Text
=
dt.
Rows
[
curRecord]
[
3
]
.
ToString
(
)
;
- txtgender.
Text
=
dt.
Rows
[
curRecord]
[
4
]
.
ToString
(
)
;
- txtaddress.
Text
=
dt.
Rows
[
curRecord]
[
5
]
.
ToString
(
)
;
- }
- private
void
btnprev_Click(
object
sender, EventArgs e)
- {
- //check if the current record is greater than zero
- if
(
curRecord >
0
)
- {
- //then decrement the current record by one
- curRecord =
curRecord -
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- }
- private
void
btnnext_Click(
object
sender, EventArgs e)
- {
- //check if the current record is not equal to the total Record minus by one
- if
(
curRecord !=
totRecord -
1
)
- {
- //increment the current record by one
- curRecord =
curRecord +
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- }
- private
void
btnfirst_Click(
object
sender, EventArgs e)
- {
- //check if the current record is not equal to zero
- if
(
curRecord !=
0
)
- //set the current record to zero
- curRecord =
0
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- private
void
btnlast_Click(
object
sender, EventArgs e)
- {
- //check if the current Record is not Equal to the total record
- if
(
curRecord !=
totRecord)
- //set the current record equal to total record
- curRecord =
totRecord -
1
;
- //call the Navaigate sub procedure
- Navigate(
)
;
- //set the record value
- lblrecord.
Text
=
curRecord +
1
+
" of "
+
totRecord;
- }
- }
- }
Download
You must upgrade your account or reply in the thread to view the hidden content.