yetg
Virtual Environment Auditor
LEVEL 1
100 XP
Good Day!!!
In this tutorial, we are going to learn on How To Change Password Using PHP. In any Web Application, changing password is important feature.
Now, let us create a change password using PHP script.
database name - change_password
old password : change_password
Directions:
For CSS Codes
For HTML Codes
For PHP Codes
Full Source Code
So what can you say about this work? Share your thoughts in the comment section below. Practice Coding. Thank you.
Download
In this tutorial, we are going to learn on How To Change Password Using PHP. In any Web Application, changing password is important feature.
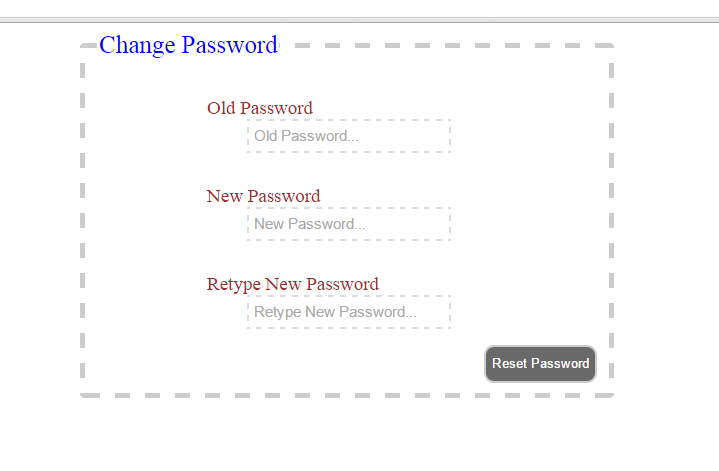
Now, let us create a change password using PHP script.
database name - change_password
old password : change_password
Directions:
For CSS Codes
- <style type=
"text/css"
>
- fieldset {
- width
:
500px
;
- border
:
5px
dashed
#CCCCCC
;
- margin
:
0
auto
;
- border-radius
:
5px
;
- }
- legend {
- color
:
blue
;
- font-size
:
25px
;
- }
- dl {
- float
:
right
;
- width
:
390px
;
- }
- dt {
- width
:
180px
;
- color
:
brown
;
- font-size
:
19px
;
- }
- dd {
- width
:
200px
;
- float
:
left
;
- }
- dd input {
- width
:
200px
;
- border
:
2px
dashed
#DDD
;
- font-size
:
15px
;
- text-indent
:
5px
;
- height
:
28px
;
- }
- .btn
{
- color
:
#fff
;
- background-color
:
dimgrey
;
- height
:
38px
;
- border
:
2px
solid
#CCC
;
- border-radius
:
10px
;
- float
:
right
;
- }
- </style>
For HTML Codes
- <fieldset
>
- <legend
>
Change Password</
legend
>
- <form
method
=
"post"
>
- <dl
>
- <dt
>
- Old Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"old_pass"
placeholder=
"Old Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <dl
>
- <dt
>
- New Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"new_pass"
placeholder=
"New Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <dl
>
- <dt
>
- Retype New Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"re_pass"
placeholder=
"Retype New Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <p
align
=
"center"
>
- <input
type
=
"submit"
class
=
"btn"
value
=
"Reset Password"
name
=
"re_password"
/
>
- </
p
>
- </
form
>
- </
fieldset
>
For PHP Codes
- <?php
- $conn_db
=
mysql_connect
(
"localhost"
,
"root"
,
""
)
or die
(
)
;
- $sel_db
=
mysql_select_db
(
"change_password"
,
$conn_db
)
or die
(
)
;
- if
(
isset
(
$_POST
[
're_password'
]
)
)
- {
- $old_pass
=
$_POST
[
'old_pass'
]
;
- $new_pass
=
$_POST
[
'new_pass'
]
;
- $re_pass
=
$_POST
[
're_pass'
]
;
- $chg_pwd
=
mysql_query
(
"select * from users where id='1'"
)
;
- $chg_pwd1
=
mysql_fetch_array
(
$chg_pwd
)
;
- $data_pwd
=
$chg_pwd1
[
'password'
]
;
- if
(
$data_pwd
==
$old_pass
)
{
- if
(
$new_pass
==
$re_pass
)
{
- $update_pwd
=
mysql_query
(
"update users set password='$new_pass
' where id='1'"
)
;
- echo
"<script>alert('Update Sucessfully'); window.location='index.php'</script>"
;
- }
- else
{
- echo
"<script>alert('Your new and Retype Password is not match'); window.location='index.php'</script>"
;
- }
- }
- else
- {
- echo
"<script>alert('Your old password is wrong'); window.location='index.php'</script>"
;
- }
}
- ?>
Full Source Code
- <!DOCTYPE html>
- <html
>
- <head
>
- <title
>
Change Password</
title
>
- <style
type
=
"text/css"
>
- fieldset {
- width:500px;
- border:5px dashed #CCCCCC;
- margin:0 auto;
- border-radius:5px;
- }
- legend {
- color: blue;
- font-size: 25px;
- }
- dl {
- float: right;
- width: 390px;
- }
- dt {
- width: 180px;
- color: brown;
- font-size: 19px;
- }
- dd {
- width:200px;
- float:left;
- }
- dd input {
- width: 200px;
- border: 2px dashed #DDD;
- font-size: 15px;
- text-indent: 5px;
- height: 28px;
- }
- .btn {
- color: #fff;
- background-color: dimgrey;
- height: 38px;
- border: 2px solid #CCC;
- border-radius: 10px;
- float: right;
- }
- </
style
>
- </
head
>
- <body
>
- <fieldset
>
- <legend
>
Change Password</
legend
>
- <?php
- $conn_db =
mysql_connect(
"localhost"
,"root"
,""
)
or die(
)
;
- $sel_db =
mysql_select_db(
"change_password"
,$conn_db)
or die(
)
;
- if(
isset(
$_POST[
're_password'
]
)
)
- {
- $old_pass=
$_POST[
'old_pass'
]
;
- $new_pass=
$_POST[
'new_pass'
]
;
- $re_pass=
$_POST[
're_pass'
]
;
- $chg_pwd=
mysql_query(
"select * from users where id='1'"
)
;
- $chg_pwd1=
mysql_fetch_array(
$chg_pwd)
;
- $data_pwd=
$chg_pwd1[
'password'
]
;
- if(
$data_pwd==
$old_pass)
{
- if(
$new_pass==
$re_pass)
{
- $update_pwd=
mysql_query(
"update users set password='$new_pass' where id='1'"
)
;
- echo "<script>
alert('Update Sucessfully'); window.location='index.php'</
script
>
";
- }
- else{
- echo "<script
>
alert('Your new and Retype Password is not match'); window.location='index.php'</
script
>
";
- }
- }
- else
- {
- echo "<script
>
alert('Your old password is wrong'); window.location='index.php'</
script
>
";
- }}
- ?>
- <form
method
=
"post"
>
- <dl
>
- <dt
>
- Old Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"old_pass"
placeholder=
"Old Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <dl
>
- <dt
>
- New Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"new_pass"
placeholder=
"New Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <dl
>
- <dt
>
- Retype New Password
- </
dt
>
- <dd
>
- <input
type
=
"password"
name
=
"re_pass"
placeholder=
"Retype New Password..."
value
=
""
required /
>
- </
dd
>
- </
dl
>
- <p
align
=
"center"
>
- <input
type
=
"submit"
class
=
"btn"
value
=
"Reset Password"
name
=
"re_password"
/
>
- </
p
>
- </
form
>
- </
fieldset
>
- </
body
>
- </
html
>
So what can you say about this work? Share your thoughts in the comment section below. Practice Coding. Thank you.
Download
You must upgrade your account or reply in the thread to view the hidden content.