Hatsune
Battle Tactician
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
In this tutorial, i will going to teach you how to create a folder and its content using C#. I always got an error in creating this program prompted as "Directory is not empty" and it cannot be deleted. So, I came up this program and studied again the possible code to fix this.
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Delete Folder.
2. Next, add only one Button named Button1 and labeled it as "Delete Folder". Add also TextBox named TextBox1 for our inputting the path of the folder that you want to delete. You must design your interface like this:
3. To achieve that, first of all we need to include the following statement in the program code:
using System.IO - This namespace has to precede the whole program code as it is higher in hierarchy for DirectoryInfo. In Fact, this is the concept of object oriented programming where DirectoryInfo is part of the namespace System.IO.
4. Now, to avoid an error saying Directory is not empty when we delete a folder, we will create a method named DeleteDirectory with string target_dir as String as parameter.
The syntax Directory.
Delete
(
target, true
)
;
will delete all the contents of the folder directory. If false, then the content will not be deleted.
5. Now to delete the folder, we will now code for Button1. We will just use the method above.
We have created an instance of DirectoryInfo that holds the path inputted in textbox1. If the directory is existed, then we have used the method DeleteDirectory and its textbox1 as the parameter. Otherwise, it will prompt the user that the path was not found.
Press F5 to run the program.
Output:
Full source code:
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
Now, let's start this tutorial!
1. Let's start with creating a Windows Form Application in C# for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application and name your project as Delete Folder.
2. Next, add only one Button named Button1 and labeled it as "Delete Folder". Add also TextBox named TextBox1 for our inputting the path of the folder that you want to delete. You must design your interface like this:
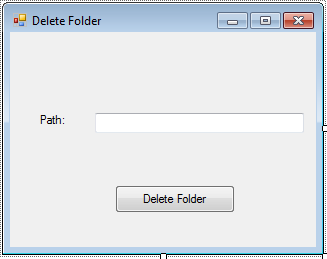
3. To achieve that, first of all we need to include the following statement in the program code:
using System.IO - This namespace has to precede the whole program code as it is higher in hierarchy for DirectoryInfo. In Fact, this is the concept of object oriented programming where DirectoryInfo is part of the namespace System.IO.
- using
System
;
- using
System.Collections.Generic
;
- using
System.ComponentModel
;
- using
System.Data
;
- using
System.Drawing
;
- using
System.Text
;
- using
System.Windows.Forms
;
- using
System.IO
;
4. Now, to avoid an error saying Directory is not empty when we delete a folder, we will create a method named DeleteDirectory with string target_dir as String as parameter.
- public
static
void
DeleteDirectory(
string
target)
- {
- string
[
]
files =
Directory.
GetFiles
(
target)
;
- string
[
]
dir =
Directory.
GetDirectories
(
target)
;
- foreach
(
string
file in
files)
- {
- File.
SetAttributes
(
file, FileAttributes.
Normal
)
;
- File.
Delete
(
file)
;
- }
- foreach
(
string
dir in
dir)
- {
- DeleteDirectory(
dir)
;
- }
- Directory.
Delete
(
target, true
)
;
- }
The syntax Directory.
Delete
(
target, true
)
;
will delete all the contents of the folder directory. If false, then the content will not be deleted.
5. Now to delete the folder, we will now code for Button1. We will just use the method above.
- private
void
Button1_Click(
object
sender, EventArgs e)
- {
- DirectoryInfo di =
new
DirectoryInfo(
TextBox1.
Text
)
;
- if
(
di.
Exists
)
- {
- DeleteDirectory(
TextBox1.
Text
)
;
- MessageBox.
Show
(
"The directory was deleted successfully"
)
;
- TextBox1.
Clear
(
)
;
- }
- else
- {
- MessageBox.
Show
(
" Path is not found!"
, "Error"
)
;
- TextBox1.
Clear
(
)
;
- }
- }
We have created an instance of DirectoryInfo that holds the path inputted in textbox1. If the directory is existed, then we have used the method DeleteDirectory and its textbox1 as the parameter. Otherwise, it will prompt the user that the path was not found.
Press F5 to run the program.
Output:
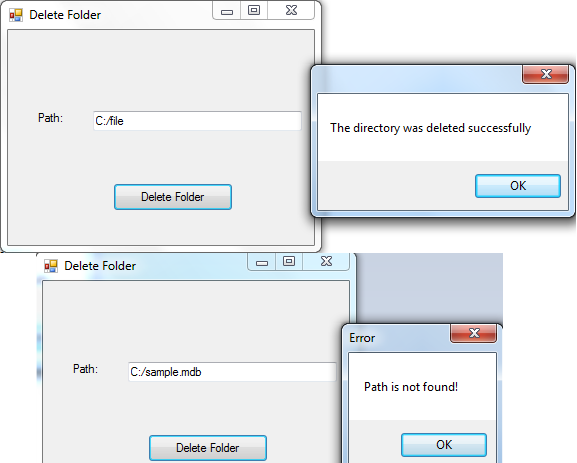
Full source code:
- using
System
;
- using
System.Collections.Generic
;
- using
System.ComponentModel
;
- using
System.Data
;
- using
System.Drawing
;
- using
System.Text
;
- using
System.Windows.Forms
;
- using
System.IO
;
- namespace
Delete_Folder
- {
- public
partial
class
Form1 :
Form
- {
- public
Form1(
)
- {
- InitializeComponent(
)
;
- }
- public
static
void
DeleteDirectory(
string
target)
- {
- string
[
]
files =
Directory.
GetFiles
(
target)
;
- string
[
]
dir =
Directory.
GetDirectories
(
target)
;
- foreach
(
string
file in
files)
- {
- File.
SetAttributes
(
file, FileAttributes.
Normal
)
;
- File.
Delete
(
file)
;
- }
- foreach
(
string
dir in
dir)
- {
- DeleteDirectory(
dir)
;
- }
- Directory.
Delete
(
target, true
)
;
- }
- private
void
Button1_Click(
object
sender, EventArgs e)
- {
- DirectoryInfo di =
new
DirectoryInfo(
TextBox1.
Text
)
;
- if
(
di.
Exists
)
- {
- DeleteDirectory(
TextBox1.
Text
)
;
- MessageBox.
Show
(
"The directory was deleted successfully"
)
;
- TextBox1.
Clear
(
)
;
- }
- else
- {
- MessageBox.
Show
(
" Path is not found!"
, "Error"
)
;
- TextBox1.
Clear
(
)
;
- }
- }
- }
- }
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
You must upgrade your account or reply in the thread to view the hidden content.