sedheali5
DAO Governance Leader
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
500 XP
Operating System
Android
Creating Score Manager
We will now create the scoring of the game. This will track down the score of the player every time the enemy is defeated. To do that go to GameObject and select UI, then choose Text. After creating the text set the components as shown below.
Score
Next create another UI Text as a child of the component above. Then set the components as shown below.
Score Text
After creating the Score text we will now create the Highscore UI text. Same as what you did to the UI Score, create a text element and set the component as show below.
Highscore
Then create a child UI Text and set the component as shown below also.
Creating Destroy boundary
We will create now the destroy boundary. This will take care of all the outside boundary objects within the screen. To do that simple create two GameObjects and add a Box Collider 2D to each GameObject. After that go to the Scripts directory then create a new directory namely Boundary. Then create a C# script called Boundary. Next write these block of code inside the class:
After creating the script attach the scripts to the GameObject inspector and set the components as shown below.
Creating Gameplay Controller
Now we will create the Gameplay Controller. This will be the game mechanics of the game. It will handle the flow of the game to make it playable. Create a GameObject then name it as Gameplay Controller. Then create a script and save it to Game Controllers folder as GameplayController.
Write these important variables to make the game work properly:
Then write the rest of the codes
After creating the script attach it to the Gameplay Controller component. And then attach all the needed components in the Inspector of GameplayController script.
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Download
Android
Creating Score Manager
We will now create the scoring of the game. This will track down the score of the player every time the enemy is defeated. To do that go to GameObject and select UI, then choose Text. After creating the text set the components as shown below.
Score
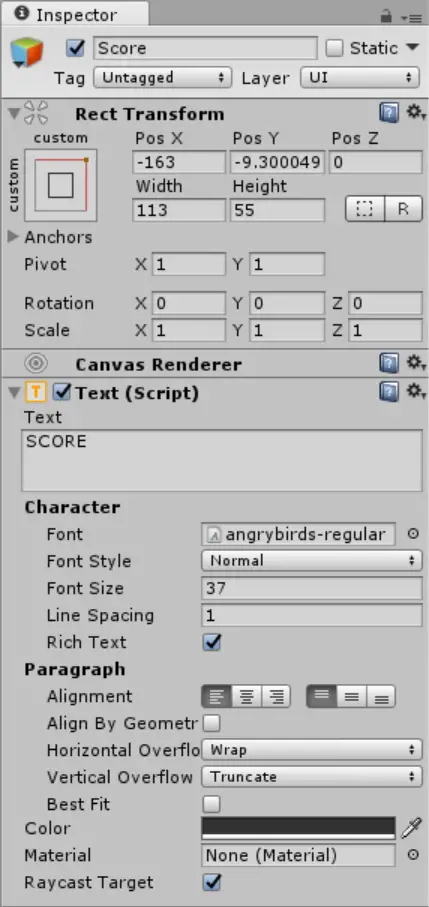
Next create another UI Text as a child of the component above. Then set the components as shown below.
Score Text
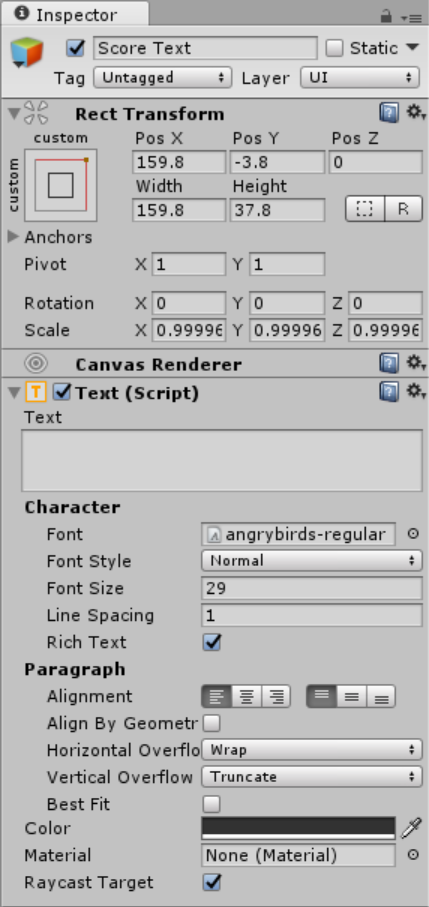
After creating the Score text we will now create the Highscore UI text. Same as what you did to the UI Score, create a text element and set the component as show below.
Highscore
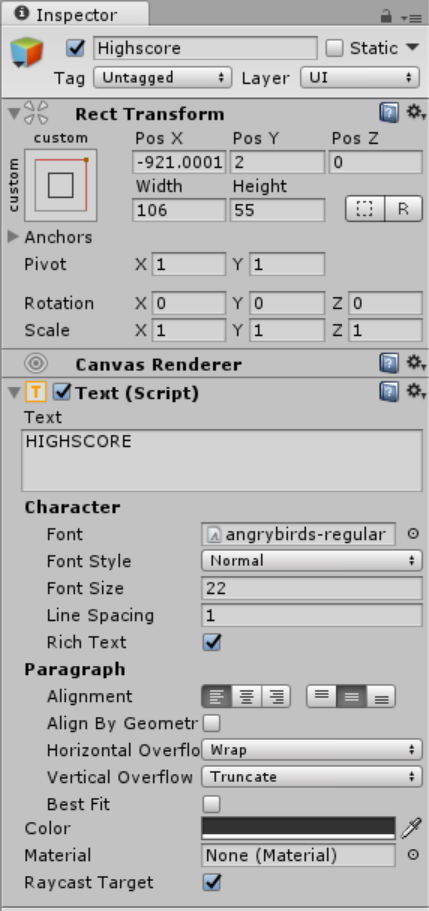
Then create a child UI Text and set the component as shown below also.
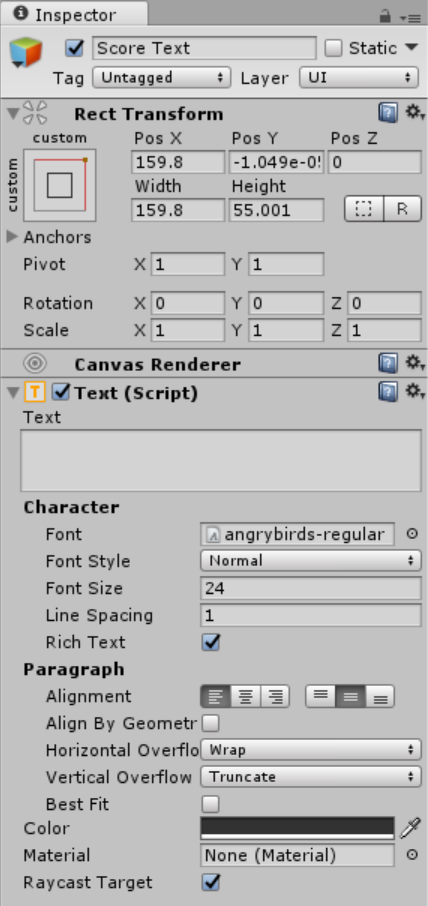
Creating Destroy boundary
We will create now the destroy boundary. This will take care of all the outside boundary objects within the screen. To do that simple create two GameObjects and add a Box Collider 2D to each GameObject. After that go to the Scripts directory then create a new directory namely Boundary. Then create a C# script called Boundary. Next write these block of code inside the class:
- // Use this for initialization
- void
Start (
)
{
- }
- // Update is called once per frame
- void
Update (
)
{
- }
- void
OnTriggerExit2D(
Collider2D collider)
{
- if
(
collider.
CompareTag
(
"Player Bullet"
)
||
collider.
CompareTag
(
"Object"
)
||
collider.
CompareTag
(
"Enemy"
)
)
{
- Destroy (
collider.
gameObject
)
;
- }
- }
After creating the script attach the scripts to the GameObject inspector and set the components as shown below.
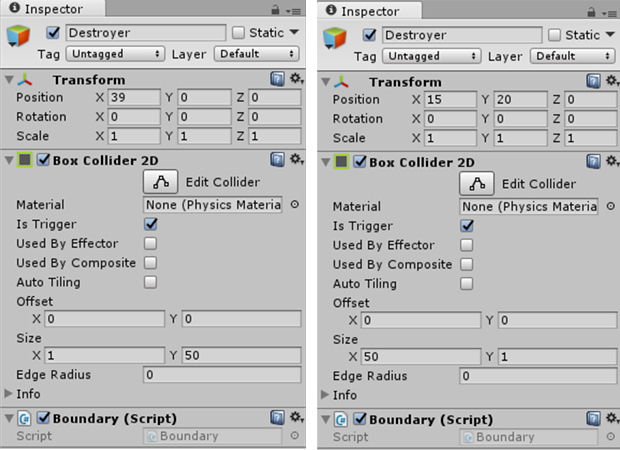
Creating Gameplay Controller
Now we will create the Gameplay Controller. This will be the game mechanics of the game. It will handle the flow of the game to make it playable. Create a GameObject then name it as Gameplay Controller. Then create a script and save it to Game Controllers folder as GameplayController.
Write these important variables to make the game work properly:
- public
static
GameplayController instance;
- public
bool
gameInProgress;
- public
Transform player;
- public
CameraFollow camera;
- public
Text scoreText, shotText, highscore;
- public
GameObject gameOverPanel, gameWinPanel, pausePanel;
- [
HideInInspector]
- public
bool
lastShot;
- private
bool
gameFinished, checkGameStatus;
- private
List<
GameObject>
enemies;
- private
List<
GameObject>
objects;
- private
float
timeAfterLastShot, distance, time, timeSinceStartedShot;
- private
int
prevLevel;
Then write the rest of the codes
- void
Awake(
)
{
- CreateInstance (
)
;
- }
- // Use this for initialization
- void
Start (
)
{
- InitializeVariables (
)
;
- if
(
GameController.
instance
!=
null
&&
MusicController.
instance
!=
null
)
{
- if
(
GameController.
instance
.
isMusicOn
)
{
- MusicController.
instance
.
GameplaySound
(
)
;
- }
else
{
- MusicController.
instance
.
StopAllSounds
(
)
;
- }
- }
- }
- // Update is called once per frame
- void
Update (
)
{
- if
(
gameInProgress)
{
- GameIsOnPlay (
)
;
- DistanceBetweenCannonAndBullet (
)
;
- }
- if
(
GameController.
instance
!=
null
)
{
- UpdateGameplayController (
)
;
- }
- }
- void
CreateInstance(
)
{
- if
(
instance ==
null
)
{
- instance =
this
;
- }
- }
- void
UpdateGameplayController(
)
{
- scoreText.
text
=
GameController.
instance
.
score
.
ToString
(
"N0"
)
;
- shotText.
text
=
"X"
+
PlayerBullet (
)
;
- }
- void
InitializeVariables(
)
{
- gameInProgress =
true
;
- enemies =
new
List<
GameObject>
(
GameObject.
FindGameObjectsWithTag
(
"Enemy"
)
)
;
- objects =
new
List<
GameObject>
(
GameObject.
FindGameObjectsWithTag
(
"Object"
)
)
;
- distance =
10f;
- if
(
GameController.
instance
!=
null
)
{
- GameController.
instance
.
score
=
0
;
- prevLevel =
GameController.
instance
.
currentLevel
;
- highscore.
transform
.
GetChild
(
0
)
.
transform
.
GetComponent
<
Text>
(
)
.
text
=
GameController.
instance
.
highscore
[
GameController.
instance
.
currentLevel
-
1
]
.
ToString
(
"N0"
)
;
- if
(
GameController.
instance
.
highscore
[
GameController.
instance
.
currentLevel
-
1
]
>
0
)
{
- highscore.
gameObject
.
SetActive
(
true
)
;
- }
- }
- }
- void
GameIsOnPlay(
)
{
- /*if (PlayerBullet () == 0) {
- timeAfterLastShot += Time.deltaTime;
- camera.isFollowing = false;
- if (timeAfterLastShot > 2f) {
- if (AllStopMoving () && AllEnemiesDestroyed ()) {
- if (!gameFinished) {
- gameFinished = true;
- Debug.Log ("Hello World");
- }
- } else if (AllStopMoving () && !AllEnemiesDestroyed ()) {
- if (!gameFinished) {
- gameFinished = true;
- Debug.Log ("Hi World");
- }
- }
- }
- }*/
- if
(
checkGameStatus)
{
- timeAfterLastShot +=
Time.
deltaTime
;
- if
(
timeAfterLastShot >
2f)
{
- if
(
AllStopMoving (
)
||
Time.
time
-
timeSinceStartedShot >
8f)
{
- if
(
AllEnemiesDestroyed (
)
)
{
- if
(
!
gameFinished)
{
- gameFinished =
true
;
- GameWin (
)
;
- timeAfterLastShot =
0
;
- checkGameStatus =
false
;
- }
- }
else
{
- if
(
PlayerBullet (
)
==
0
)
{
- if
(
!
gameFinished)
{
- gameFinished =
true
;
- timeAfterLastShot =
0
;
- checkGameStatus =
false
;
- GameLost (
)
;
- }
- }
else
{
- checkGameStatus =
false
;
- camera.
isFollowing
=
false
;
- timeAfterLastShot =
0
;
- }
- }
- }
- }
- }
- }
- void
GameWin(
)
{
- if
(
GameController.
instance
!=
null
&&
MusicController.
instance
!=
null
)
{
- if
(
GameController.
instance
.
isMusicOn
)
{
- AudioSource.
PlayClipAtPoint
(
MusicController.
instance
.
winSound
, Camera.
main
.
transform
.
position
)
;
- }
- if
(
GameController.
instance
.
score
>
GameController.
instance
.
highscore
[
GameController.
instance
.
currentLevel
-
1
]
)
{
- GameController.
instance
.
highscore
[
GameController.
instance
.
currentLevel
-
1
]
=
GameController.
instance
.
score
;
- }
- highscore.
text
=
GameController.
instance
.
highscore
[
GameController.
instance
.
currentLevel
]
.
ToString
(
"N0"
)
;
- int
level =
GameController.
instance
.
currentLevel
;
- level++;
- if
(
!
(
level-
1
>=
GameController.
instance
.
levels
.
Length
)
)
{
- GameController.
instance
.
levels
[
level -
1
]
=
true
;
- }
- GameController.
instance
.
Save
(
)
;
- GameController.
instance
.
currentLevel
=
level;
- }
- gameWinPanel.
SetActive
(
true
)
;
- }
- void
GameLost(
)
{
- if
(
GameController.
instance
!=
null
&&
MusicController.
instance
!=
null
)
{
- if
(
GameController.
instance
.
isMusicOn
)
{
- AudioSource.
PlayClipAtPoint
(
MusicController.
instance
.
loseSound
, Camera.
main
.
transform
.
position
)
;
- }
- }
- gameOverPanel.
SetActive
(
true
)
;
- }
- public
int
PlayerBullet(
)
{
- int
playerBullet =
GameObject.
FindGameObjectWithTag
(
"Player"
)
.
transform
.
GetChild
(
0
)
.
transform
.
GetComponent
<
Cannon>
(
)
.
shot
;
- return
playerBullet;
- }
- private
bool
AllEnemiesDestroyed(
)
{
- return
enemies.
All
(
x =>
x ==
null
)
;
- }
- private
bool
AllStopMoving(
)
{
- foreach
(
var
item in
objects.
Union
(
enemies)
)
{
- if
(
item !=
null
&&
item.
GetComponent
<
Rigidbody2D>
(
)
.
velocity
.
sqrMagnitude
>
0
)
{
- return
false
;
- }
- }
- return
true
;
- }
- void
DistanceBetweenCannonAndBullet(
)
{
- GameObject[
]
bullet =
GameObject.
FindGameObjectsWithTag
(
"Player Bullet"
)
;
- foreach
(
GameObject distanceToBullet in
bullet)
{
- if
(
!
distanceToBullet.
transform
.
GetComponent
<
CannonBullet>
(
)
.
isIdle
)
{
- if
(
distanceToBullet.
transform
.
position
.
x
-
player.
position
.
x
>
distance)
{
- camera.
isFollowing
=
true
;
- checkGameStatus =
true
;
- timeSinceStartedShot =
Time.
time
;
- TimeSinceShot (
)
;
- camera.
target
=
distanceToBullet.
transform
;
- }
else
{
- if
(
PlayerBullet(
)
==
0
)
{
- camera.
isFollowing
=
true
;
- checkGameStatus =
true
;
- timeSinceStartedShot =
Time.
time
;
- TimeSinceShot (
)
;
- camera.
target
=
distanceToBullet.
transform
;
- }
- }
- }
- }
- /*if (GameObject.FindGameObjectWithTag ("Player Bullet") != null) {
- if (!GameObject.FindGameObjectWithTag ("Player Bullet").transform.GetComponent<CannonBullet> ().isIdle) {
- Transform distanceToBullet = GameObject.FindGameObjectWithTag ("Player Bullet").transform;
- if (distanceToBullet.position.x - player.position.x > distance) {
- camera.isFollowing = true;
- checkGameStatus = true;
- TimeSinceShot ();
- camera.target = distanceToBullet;
- }
- }
- }*/
- }
- void
TimeSinceShot(
)
{
- time +=
Time.
deltaTime
;
- if
(
time >
3f)
{
- time =
0f;
- GameObject.
FindGameObjectWithTag
(
"Player Bullet"
)
.
transform
.
GetComponent
<
CannonBullet>
(
)
.
isIdle
=
true
;
- }
- }
- public
void
RestartGame(
)
{
- SceneManager.
LoadScene
(
SceneManager.
GetActiveScene
(
)
.
buildIndex
)
;
- if
(
GameController.
instance
!=
null
)
{
- GameController.
instance
.
currentLevel
=
prevLevel;
- }
- }
- public
void
BackToLevelMenu(
)
{
- if
(
GameController.
instance
!=
null
&&
MusicController.
instance
!=
null
)
{
- if
(
GameController.
instance
.
isMusicOn
)
{
- MusicController.
instance
.
PlayBgMusic
(
)
;
- }
else
{
- MusicController.
instance
.
StopAllSounds
(
)
;
- }
- }
- SceneManager.
LoadScene
(
"Level Menu"
)
;
- Time.
timeScale
=
1
;
- }
- public
void
ContinueGame(
)
{
- if
(
GameController.
instance
!=
null
)
{
- SceneManager.
LoadScene
(
"Level "
+
GameController.
instance
.
currentLevel
)
;
- }
- }
- public
void
PauseGame(
)
{
- if
(
gameInProgress)
{
- gameInProgress =
false
;
- Time.
timeScale
=
0
;
- pausePanel.
SetActive
(
true
)
;
- }
- }
- public
void
ResumeGame(
)
{
- Time.
timeScale
=
1
;
- gameInProgress =
true
;
- pausePanel.
SetActive
(
false
)
;
- }
After creating the script attach it to the Gameplay Controller component. And then attach all the needed components in the Inspector of GameplayController script.
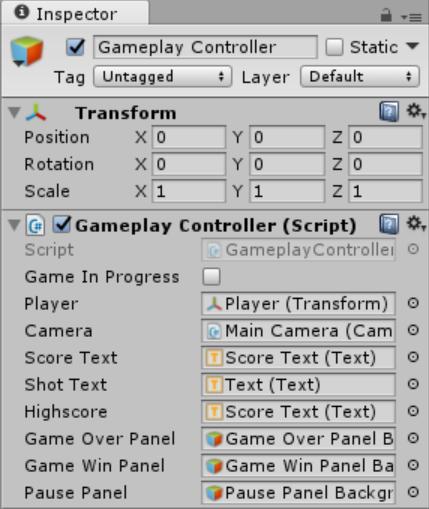
Note: Due to the size or complexity of this submission, the author has submitted it as a .zip file to shorten your download time. After downloading it, you will need a program like Winzip to decompress it.
Virus note: All files are scanned once-a-day by SourceCodester.com for viruses, but new viruses come out every day, so no prevention program can catch 100% of them.
FOR YOUR OWN SAFETY, PLEASE:
1. Re-scan downloaded files using your personal virus checker before using it.
2. NEVER, EVER run compiled files (.exe's, .ocx's, .dll's etc.)--only run source code.
Download
You must upgrade your account or reply in the thread to view hidden text.