puffy009
Freelance Platform Wizard
LEVEL 1
300 XP
Getting Started
I've used CDN of Bootstrap, Vue.js and Axios.js in this tutorial, so you need internet connection for them to work.
Creating our Database
1. Open phpMyAdmin.
2. Click databases, create a database and name it as vuealert.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
style.css
This is our customize CSS.
index.php
This contains our form to trigger our alert and also contains our sample table.
app.js
This contains our vue.js codes.
action.php
Lastly, this our PHP code which contains our validations to trigger alerts.
That ends this tutorial. Happy Coding :)
Download
I've used CDN of Bootstrap, Vue.js and Axios.js in this tutorial, so you need internet connection for them to work.
Creating our Database
1. Open phpMyAdmin.
2. Click databases, create a database and name it as vuealert.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
- CREATE
TABLE
`members`
(
- `memid`
INT
(
11
)
NOT
NULL
AUTO_INCREMENT
,
- `email`
VARCHAR
(
60
)
NOT
NULL
,
- `password`
VARCHAR
(
50
)
NOT
NULL
,
- PRIMARY
KEY
(
`memid`
)
- )
ENGINE=
InnoDB DEFAULT
CHARSET=
latin1;
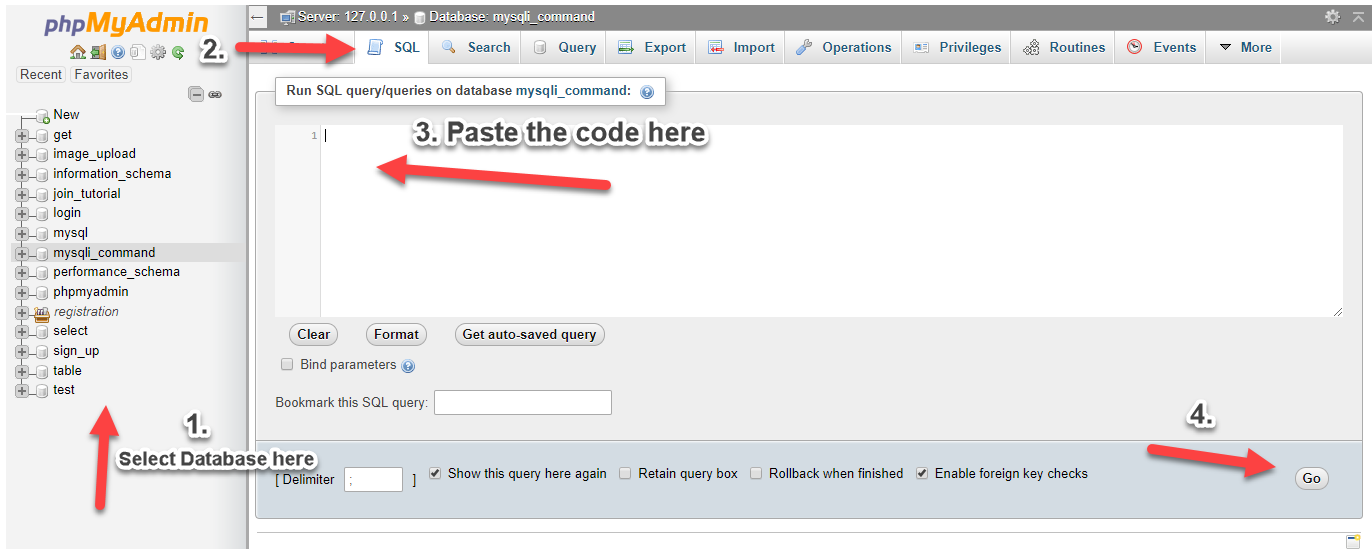
style.css
This is our customize CSS.
- .topcorner{
- position
:
absolute
;
- top
:
5px
;
- right
:
5px
;
- }
- .alert_danger
{
- padding
:
15px
;
- background-color
:
#f44336
;
- color
:
white
;
- }
- .alert_success
{
- padding
:
15px
;
- background-color
:
#4CAF50
;
- color
:
white
;
- }
- .closebutton
{
- margin-left
:
15px
;
- color
:
white
;
- font-weight
:
bold
;
- float
:
right
;
- font-size
:
22px
;
- line-height
:
20px
;
- cursor
:
pointer
;
- transition
:
0.3s
;
- }
- .closebutton
:
hover
{
- color
:
black
;
- }
index.php
This contains our form to trigger our alert and also contains our sample table.
- <!DOCTYPE html>
- <html
>
- <head
>
- <title
>
Create an Alert using Vue.js with PHP</
title
>
- <link
rel
=
"stylesheet"
href
=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"
/
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.10/vue.min.js"
></
script
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/axios/0.17.1/axios.min.js"
></
script
>
- <link
rel
=
"stylesheet"
type
=
"text/css"
href
=
"style.css"
>
- </
head
>
- <body
>
- <div
id
=
"alert"
>
- <div
class
=
"topcorner alert_danger"
v-if=
"isError"
>
- <span
class
=
"closebutton"
@click=
"clearMessage();"
>
×
</
span
>
- <span
class
=
"glyphicon glyphicon-alert"
></
span
>
{{ responseMessage }}
- </
div
>
- <div
class
=
"topcorner alert_success"
v-if=
"isSuccess"
>
- <span
class
=
"closebutton"
@click=
"clearMessage();"
>
×
</
span
>
- <span
class
=
"glyphicon glyphicon-check-square-o"
></
span
>
{{ responseMessage }}
- </
div
>
- <div
class
=
"container"
>
- <h1
class
=
"page-header text-center"
>
Create an Alert using Vue.js with PHP</
h1
>
- <div
class
=
"col-md-4"
>
- <div
class
=
"form-group"
>
- <label
>
Email:</
label
>
- <input
type
=
"text"
class
=
"form-control"
v-model=
"newMember.email"
v-on:keyup=
"keymonitor"
>
- </
div
>
- <div
class
=
"form-group"
>
- <label
>
Password:</
label
>
- <input
type
=
"text"
class
=
"form-control"
v-model=
"newMember.password"
v-on:keyup=
"keymonitor"
>
- </
div
>
- <button
class
=
"btn btn-primary"
@click=
"insertMember();"
><span
class
=
"glyphicon glyphicon-floppy-disk"
></
span
>
Save</
button
>
<button
class
=
"btn btn-danger"
@click=
"clearForm();"
><span
class
=
"glyphicon glyphicon-refresh"
></
span
>
Clear</
button
>
- </
div
>
- <div
class
=
"col-md-8"
>
- <table
class
=
"table table-bordered table-striped"
>
- <thead
>
- <th
>
Member ID</
th
>
- <th
>
Email</
th
>
- <th
>
Password</
th
>
- </
thead
>
- <tbody
>
- <tr
v-for
=
"member in members"
>
- <td
>
{{ member.memid }}</
td
>
- <td
>
{{ member.email }}</
td
>
- <td
>
{{ member.password }}</
td
>
- </
tr
>
- </
tbody
>
- </
table
>
- </
div
>
- </
div
>
- </
div
>
- <script
src
=
"app.js"
></
script
>
- </
body
>
- </
html
>
app.js
This contains our vue.js codes.
- var
app =
new
Vue(
{
- el:
'#alert'
,
- data:
{
- newMember:
{
email:
''
,
password:
''
}
,
- alertMessage:
false
,
- isSuccess:
false
,
- isError:
false
,
- responseMessage:
""
,
- members:
[
]
- }
,
- mounted:
function
(
)
{
- this
.fetchMembers
(
)
;
- }
,
- methods:
{
- keymonitor:
function
(
event)
{
- if
(
event.key
==
"Enter"
)
{
- app.insertMember
(
)
;
- }
- }
,
- fetchMembers:
function
(
)
{
- axios.post
(
'action.php'
)
- .then
(
function
(
response)
{
- app.members
=
response.data
.members
;
- }
)
;
- }
,
- insertMember:
function
(
)
{
- var
memberForm =
app.toFormData
(
app.newMember
)
;
- axios.post
(
'action.php?action=add'
,
memberForm)
- .then
(
function
(
response)
{
- console.log
(
response)
;
- if
(
response.data
.error
)
{
- app.alertMessage
=
true
;
- app.isError
=
true
;
- app.isSuccess
=
false
;
- app.responseMessage
=
response.data
.message
;
- setTimeout(
function
(
)
{
- app.clearMessage
(
)
;
- }
,
3000
)
;
- }
- else
{
- app.isSuccess
=
true
;
- app.isError
=
false
;
- app.alertMessage
=
true
;
- app.responseMessage
=
response.data
.message
;
- app.newMember
=
{
email:
''
,
password:
''
}
;
- app.fetchMembers
(
)
;
- setTimeout(
function
(
)
{
- app.clearMessage
(
)
;
- }
,
3000
)
;
- }
- }
)
;
- }
,
- toFormData:
function
(
obj)
{
- var
form_data =
new
FormData(
)
;
- for
(
var
key in
obj)
{
- form_data.append
(
key,
obj[
key]
)
;
- }
- return
form_data;
- }
,
- clearMessage:
function
(
)
{
- app.isError
=
false
;
- app.isSuccess
=
false
;
- }
,
- clearForm:
function
(
)
{
- app.newMember
=
app.newMember
=
{
email:
''
,
password:
''
}
;
- }
- }
- }
)
;
action.php
Lastly, this our PHP code which contains our validations to trigger alerts.
- <?php
- $conn
=
new
mysqli(
"localhost"
,
"root"
,
""
,
"vuealert"
)
;
- if
(
$conn
->
connect_error
)
{
- die
(
"Connection failed: "
.
$conn
->
connect_error
)
;
- }
- $out
=
array
(
'error'
=>
false
)
;
- $action
=
"show"
;
- if
(
isset
(
$_GET
[
'action'
]
)
)
{
- $action
=
$_GET
[
'action'
]
;
- }
- if
(
$action
==
'show'
)
{
- $sql
=
"select * from members"
;
- $query
=
$conn
->
query
(
$sql
)
;
- $members
=
array
(
)
;
- while
(
$row
=
$query
->
fetch_array
(
)
)
{
- array_push
(
$members
,
$row
)
;
- }
- $out
[
'members'
]
=
$members
;
- }
- if
(
$action
==
'add'
)
{
- $email
=
$_POST
[
'email'
]
;
- $password
=
$_POST
[
'password'
]
;
- if
(
$email
==
''
)
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
'Add Member Failed. Username Empty.'
;
- }
- elseif
(
!
filter_var
(
$email
,
FILTER_VALIDATE_EMAIL)
)
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
'Add Member Failed. Invalid Email Format'
;
- }
- elseif
(
$password
==
''
)
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
'Add Member Failed. Password Empty.'
;
- }
- elseif
(
!
preg_match
(
"/^[a-zA-Z_1-9]*$/"
,
$password
)
)
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
'Underscore and Special Characters not allowed in Password'
;
- }
- else
{
- $sql
=
"insert into members (email, password) values ('$email
', '$password
')"
;
- $query
=
$conn
->
query
(
$sql
)
;
- if
(
$query
)
{
- $out
[
'message'
]
=
'Member Successfully Added'
;
- }
- else
{
- $out
[
'error'
]
=
true
;
- $out
[
'message'
]
=
'Error in Adding Occured'
;
- }
- }
- }
- $conn
->
close
(
)
;
- header
(
"Content-type: application/json"
)
;
- echo
json_encode
(
$out
)
;
- die
(
)
;
- ?>
That ends this tutorial. Happy Coding :)
Download
You must upgrade your account or reply in the thread to view the hidden content.