DoughZo
Waifu Strategist
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
In this tutorial, i will teach you on how to create a program that can search and retrieve a record from an access database and vb.net.
Now, let's start this tutorial!
1. Create an access file with the table, entities, and record below. Name your access database as sample.mdb (2003 format).
2. Create a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
3. Next, add only one Button named btnFind and labeled it as "Find". This will search the inputted text in the searched textbox. Insert 4 textbox named txtSearch for the searching of student number, txtName for Student Name, txtCourse for Student course, and txtSection for student section. You must design your interface like this:
4. Create a module in your project and named it, modConnection.
Import Imports
System.
Data
.
OleDb
library. This library package is for ms access database.
In your module connection, initialize the following variables.
Now, create a method named connection to have the connection string. This will locate the sample.mdb access database that we have created earlier.
Full code of the modConnection module.
5. Back to our form, put this code for the form_load. We will call the connetion() method that we have created in our module. Because we all know that module is access throughout the entire program.
6. For the btnFind as the search button, put this code below.
We will first create a try and catch method to have the exception handling. In the try method, we will create a Boolean variable named found for finding the student number. Here, we will use the OleDbCommand with the method of connection, CommandType.Text, and CommandText, with the OleDbDataReader to execute the CommandText.
Next is to create a while statement for dr.Read to retrieve and display the data to the specified textbox, as well as making the Boolean variable found into True. Make cn.Close() in there and exit sub after finding the student number.
If the inputted student number is not in the database, put this code below.
Here is the full source code of our Form.
After that, Press F5 to run the program.
Output:
Hope this helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Download
Now, let's start this tutorial!
1. Create an access file with the table, entities, and record below. Name your access database as sample.mdb (2003 format).

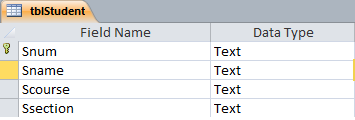
2. Create a Windows Form Application for this tutorial by following the following steps in Microsoft Visual Studio: Go to File, click New Project, and choose Windows Application.
3. Next, add only one Button named btnFind and labeled it as "Find". This will search the inputted text in the searched textbox. Insert 4 textbox named txtSearch for the searching of student number, txtName for Student Name, txtCourse for Student course, and txtSection for student section. You must design your interface like this:
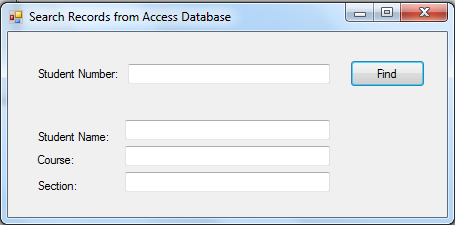
4. Create a module in your project and named it, modConnection.
Import Imports
System.
Data
.
OleDb
library. This library package is for ms access database.
In your module connection, initialize the following variables.
- Module
modConnection
- Public
cn As
New
OleDb.
OleDbConnection
- Public
cm As
New
OleDb.
OleDbCommand
- Public
dr As
OleDbDataReader
Now, create a method named connection to have the connection string. This will locate the sample.mdb access database that we have created earlier.
- Public
Sub
connection(
)
- cn =
New
OleDb.
OleDbConnection
- With
cn
- 'Provider must be Microsoft.Jet.OLEDB.4.0, find the access file, and test the connection
- .
ConnectionString
=
"Provider=Microsoft.Jet.OLEDB.4.0;Data Source="
&
Application.
StartupPath
&
"\sample.mdb"
- .
Open
(
)
- End
With
- End
Sub
Full code of the modConnection module.
- Imports
System.
Data
.
OleDb
- Module
modConnection
- Public
cn As
New
OleDb.
OleDbConnection
- Public
cm As
New
OleDb.
OleDbCommand
- Public
dr As
OleDbDataReader
- Public
Sub
connection(
)
- cn =
New
OleDb.
OleDbConnection
- With
cn
- .
ConnectionString
=
"Provider=Microsoft.Jet.OLEDB.4.0;Data Source="
&
Application.
StartupPath
&
"\sample.mdb"
- .
Open
(
)
- End
With
- End
Sub
- End
Module
5. Back to our form, put this code for the form_load. We will call the connetion() method that we have created in our module. Because we all know that module is access throughout the entire program.
- Private
Sub
Form1_Load(
ByVal
sender As
System.
Object
, ByVal
e As
System.
EventArgs
)
Handles
MyBase
.
Load
- Call
connection(
)
- End
Sub
6. For the btnFind as the search button, put this code below.
We will first create a try and catch method to have the exception handling. In the try method, we will create a Boolean variable named found for finding the student number. Here, we will use the OleDbCommand with the method of connection, CommandType.Text, and CommandText, with the OleDbDataReader to execute the CommandText.
- Dim
found As
Boolean
- Try
- cm =
New
OleDb.
OleDbCommand
- With
cm
- .
Connection
=
cn
- .
CommandType
=
CommandType.
Text
- .
CommandText
=
"SELECT * FROM tblStudent WHERE (Snum = '"
&
txtSearch.
Text
&
"')"
- dr =
.
ExecuteReader
- End
With
Next is to create a while statement for dr.Read to retrieve and display the data to the specified textbox, as well as making the Boolean variable found into True. Make cn.Close() in there and exit sub after finding the student number.
- While
dr.
Read
(
)
- txtName.
Text
=
dr(
"Sname"
)
.
ToString
- txtCourse.
Text
=
dr(
"Scourse"
)
.
ToString
- txtSection.
Text
=
dr(
"Ssection"
)
.
ToString
- found =
True
- End
While
- cn.
Close
(
)
- Exit
Sub
If the inputted student number is not in the database, put this code below.
Here is the full source code of our Form.
- Public
Class
Form1
- Private
Sub
btnFind_Click(
ByVal
sender As
System.
Object
, ByVal
e As
System.
EventArgs
)
Handles
btnFind.
Click
- Dim
found As
Boolean
- Try
- cm =
New
OleDb.
OleDbCommand
- With
cm
- .
Connection
=
cn
- .
CommandType
=
CommandType.
Text
- .
CommandText
=
"SELECT * FROM tblStudent WHERE (Snum = '"
&
txtSearch.
Text
&
"')"
- dr =
.
ExecuteReader
- End
With
- While
dr.
Read
(
)
- txtName.
Text
=
dr(
"Sname"
)
.
ToString
- txtCourse.
Text
=
dr(
"Scourse"
)
.
ToString
- txtSection.
Text
=
dr(
"Ssection"
)
.
ToString
- found =
True
- End
While
- cn.
Close
(
)
- Exit
Sub
- If
found =
False
Then
MsgBox
(
"Student ID not found."
, MsgBoxStyle.
Critical
)
- dr.
Close
(
)
- Catch
ex As
Exception
- End
Try
- End
Sub
- Private
Sub
Form1_Load(
ByVal
sender As
System.
Object
, ByVal
e As
System.
EventArgs
)
Handles
MyBase
.
Load
- Call
connection(
)
- End
Sub
- End
Class
After that, Press F5 to run the program.
Output:
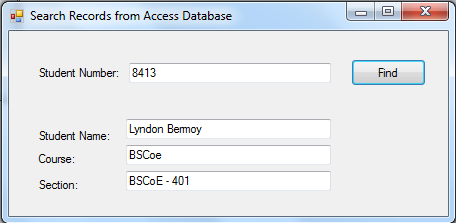
Hope this helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer
Mobile: 09079373999
Telephone: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: Bermz ISware Solutions
Subscribe at my YouTube Channel at: SerBermz
Download
You must upgrade your account or reply in the thread to view the hidden content.