socksucker
UX/UI Master
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
This tutorial tackles on how to create a search function that searches MySQL
table using Vue.js
with the help of axios.js
and PHP
. In this tutorial, you can search for either the first name or the last name of members in the members' table then it will return members that match your search.
Getting Started
I've used CDN for Bootstrap, Vue.js, and Axios.js so, you need an internet connection for them to work. And also download XAMPP
Creating our Database
Inserting Data into our Database
Next, we insert sample data into our database to use in our search.
Creating the Interface
This contains our sample table and our search input.
index.php
Creating a Script
This contains our vue.js code.
app.js
Creating the API
Lastly, this contains our PHP
code in fetching member data from our database.
action.php
DEMO
That ends this tutorial. I help you with what you are looking for and you'll find this tutorial useful for your future web application projects.
Happy Coding :)
Download
table using Vue.js
with the help of axios.js
and PHP
. In this tutorial, you can search for either the first name or the last name of members in the members' table then it will return members that match your search.
Getting Started
I've used CDN for Bootstrap, Vue.js, and Axios.js so, you need an internet connection for them to work. And also download XAMPP
Creating our Database
- Open your XAMPP's Control Panel and start "Apache" and "MySQL"
- Open PHPMyAdmin
- Click databases, create a database and name it as vuetot
- After creating a database, click the SQL
and paste the below codes. See the image below for detailed instructions.
- CREATE
TABLE
`members`
(
- `memid`
int
(
11
)
NOT
NULL
AUTO_INCREMENT
,
- `firstname`
varchar
(
30
)
NOT
NULL
,
- `lastname`
varchar
(
30
)
NOT
NULL
,
- PRIMARY KEY
(
`memid`
)
- )
ENGINE
=
InnoDB
DEFAULT
CHARSET
=
latin1;
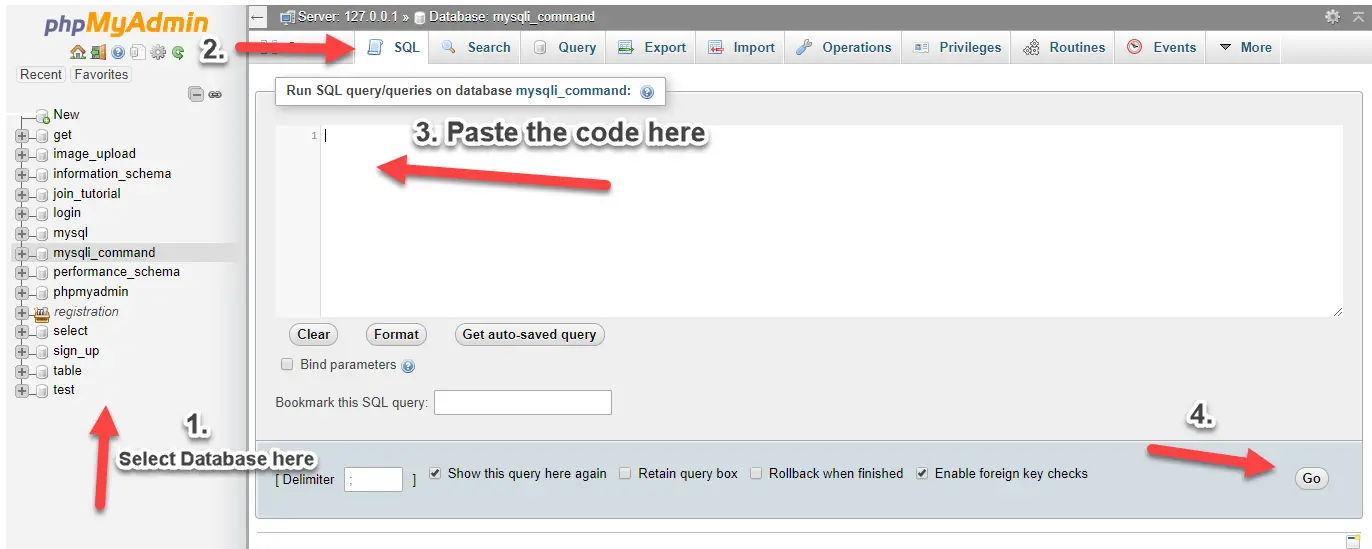
Inserting Data into our Database
Next, we insert sample data into our database to use in our search.
- Click vuetot
database that we have created earlier.
- Click SQL
and paste the following codes.
- Click Go button below.
Creating the Interface
This contains our sample table and our search input.
index.php
- <!DOCTYPE html>
- <html
>
- <head
>
- <title
>
Live Search using Vue.js with PHP</
title
>
- <link
rel
=
"stylesheet"
href
=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"
/
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.10/vue.min.js"
></
script
>
- <script
src
=
"https://cdnjs.cloudflare.com/ajax/libs/axios/0.17.1/axios.min.js"
></
script
>
- </
head
>
- <body
>
- <div
id
=
"myapp"
>
- <div
class
=
"container"
>
- <h1
class
=
"page-header text-center"
>
Live Search using Vue.js with PHP</
h1
>
- <div
class
=
"col-md-8 col-md-offset-2"
>
- <div
class
=
"row"
>
- <div
class
=
"col-md-4 col-md-offset-8"
>
- <input
type
=
"text"
class
=
"form-control"
placeholder=
"Search Firstname or Lastname"
v-on:keyup=
"searchMonitor"
v-model=
"search.keyword"
>
- </
div
>
- </
div
>
- <div
style
=
"height:5px;"
></
div
>
- <table
class
=
"table table-bordered table-striped"
>
- <thead
>
- <th
>
Firstname</
th
>
- <th
>
Lastname</
th
>
- </
thead
>
- <tbody
>
- <tr
v-if=
"noMember"
>
- <td
colspan
=
"2"
align
=
"center"
>
No member match your search</
td
>
- </
tr
>
- <tr
v-for
=
"member in members"
>
- <td
>
{{ member.firstname }}</
td
>
- <td
>
{{ member.lastname }}</
td
>
- </
tr
>
- </
tbody
>
- </
table
>
- </
div
>
- </
div
>
- </
div
>
- <script
src
=
"app.js"
></
script
>
- </
body
>
- </
html
>
Creating a Script
This contains our vue.js code.
app.js
- var
app =
new
Vue(
{
- el:
'#myapp'
,
- data:
{
- members:
[
]
,
- search:
{
keyword:
''
}
,
- noMember:
false
- }
,
- mounted:
function
(
)
{
- this
.fetchMembers
(
)
;
- }
,
- methods:
{
- searchMonitor:
function
(
)
{
- var
keyword =
app.toFormData
(
app.search
)
;
- axios.post
(
'action.php?action=search'
,
keyword)
- .then
(
function
(
response)
{
- app.members
=
response.data
.members
;
- if
(
response.data
.members
==
''
)
{
- app.noMember
=
true
;
- }
- else
{
- app.noMember
=
false
;
- }
- }
)
;
- }
,
- fetchMembers:
function
(
)
{
- axios.post
(
'action.php'
)
- .then
(
function
(
response)
{
- app.members
=
response.data
.members
;
- }
)
;
- }
,
- toFormData:
function
(
obj)
{
- var
form_data =
new
FormData(
)
;
- for
(
var
key in
obj)
{
- form_data.append
(
key,
obj[
key]
)
;
- }
- return
form_data;
- }
,
- }
- }
)
;
Creating the API
Lastly, this contains our PHP
code in fetching member data from our database.
action.php
- <?php
- $conn
=
new
mysqli(
"localhost"
,
"root"
,
""
,
"vuetot"
)
;
- if
(
$conn
->
connect_error
)
{
- die
(
"Connection failed: "
.
$conn
->
connect_error
)
;
- }
- $out
=
array
(
'error'
=>
false
)
;
- $action
=
"show"
;
- if
(
isset
(
$_GET
[
'action'
]
)
)
{
- $action
=
$_GET
[
'action'
]
;
- }
- if
(
$action
==
'show'
)
{
- $sql
=
"select * from members"
;
- $query
=
$conn
->
query
(
$sql
)
;
- $members
=
array
(
)
;
- while
(
$row
=
$query
->
fetch_array
(
)
)
{
- array_push
(
$members
,
$row
)
;
- }
- $out
[
'members'
]
=
$members
;
- }
- if
(
$action
==
'search'
)
{
- $keyword
=
$_POST
[
'keyword'
]
;
- $sql
=
"select * from members where firstname like '%$keyword
%' o
r lastname like '%$keyword
%'"
;
- $query
=
$conn
->
query
(
$sql
)
;
- $members
=
array
(
)
;
- while
(
$row
=
$query
->
fetch_array
(
)
)
{
- array_push
(
$members
,
$row
)
;
- }
- $out
[
'members'
]
=
$members
;
- }
- $conn
->
close
(
)
;
- header
(
"Content-type: application/json"
)
;
- echo
json_encode
(
$out
)
;
- die
(
)
;
- ?>
DEMO
That ends this tutorial. I help you with what you are looking for and you'll find this tutorial useful for your future web application projects.
Happy Coding :)
Download
You must upgrade your account or reply in the thread to view hidden text.