J0r12
Automated Testing Specialist
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
This is a tutorial in which we will going to create a program that has the JLabel Component using Java. The JLabel lets the user display a short text string or an image, or both. You cannot input a text on JLabel and cannot have the keyboard focus on it.
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jLabelComponent.java.
2. Import the following packages below:
3. Initialize your variable in your Main, variable frame for creating JFrame, and variable label1 to label5 for your JLabel component.
As you can see the above code,I have created 5 labels with their corresponding text and alignments. I have used the parameter of the JLabel which is the JLabel(String text, int horizontal alignment). And also you can put image on that using the ImageIcon class. The JLabel.LEFT indicates the alignment to left, JLabel.RIGHT indicates the alignment to left and JLabel.CENTER indicates the alignment to center.
Now, to set the vertical alignment of the label, we will use the setVerticalAlignment method and we will use the center orientation in the label5 variable. To have also the tooltip in the label, we will use of the setToolTipText method.
When running the program, this image will be the first to be shown.
4. To add the labels to the frame, have this code below:
5. Lastly, set the size, visibility, and the close operation of the frame. Have also the FlowLayout as the layout manager of the frame. Have this code below:
Output:
Here's the full code of this tutorial:
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
So, now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of jLabelComponent.java.
2. Import the following packages below:
- import
java.awt.*
;
// used to access the FlowLayout Class
- import
javax.swing.*
;
//used to access the JFrame and JLabel class
3. Initialize your variable in your Main, variable frame for creating JFrame, and variable label1 to label5 for your JLabel component.
- JFrame
frame =
new
JFrame
(
"JLabel Component"
)
;
- JLabel
label1 =
new
JLabel
(
"Student ID:"
, JLabel
.LEFT
)
;
- JLabel
label2 =
new
JLabel
(
"First Name:"
, JLabel
.RIGHT
)
;
- JLabel
label3 =
new
JLabel
(
"Last Name:"
, JLabel
.RIGHT
)
;
- JLabel
label4 =
new
JLabel
(
"Age:"
, JLabel
.LEFT
)
;
- JLabel
label5 =
new
JLabel
(
"Student Information"
, JLabel
.CENTER
)
;
As you can see the above code,I have created 5 labels with their corresponding text and alignments. I have used the parameter of the JLabel which is the JLabel(String text, int horizontal alignment). And also you can put image on that using the ImageIcon class. The JLabel.LEFT indicates the alignment to left, JLabel.RIGHT indicates the alignment to left and JLabel.CENTER indicates the alignment to center.
Now, to set the vertical alignment of the label, we will use the setVerticalAlignment method and we will use the center orientation in the label5 variable. To have also the tooltip in the label, we will use of the setToolTipText method.
- label5.setVerticalAlignment
(
JLabel
.CENTER
)
;
- label5.setToolTipText
(
"This is a label!"
)
;
When running the program, this image will be the first to be shown.
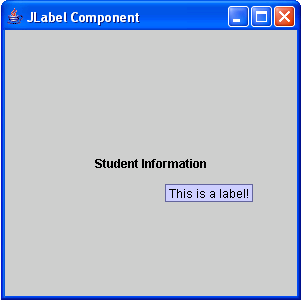
4. To add the labels to the frame, have this code below:
- frame.getContentPane
(
)
.add
(
label1)
;
- frame.getContentPane
(
)
.add
(
label2)
;
- frame.getContentPane
(
)
.add
(
label3)
;
- frame.getContentPane
(
)
.add
(
label4)
;
- frame.getContentPane
(
)
.add
(
label5)
;
5. Lastly, set the size, visibility, and the close operation of the frame. Have also the FlowLayout as the layout manager of the frame. Have this code below:
- frame.setSize
(
300
, 300
)
;
- frame.setVisible
(
true
)
;
- frame.setDefaultCloseOperation
(
JFrame
.EXIT_ON_CLOSE
)
;
- frame.getContentPane
(
)
.setLayout
(
new
FlowLayout
(
)
)
;
Output:
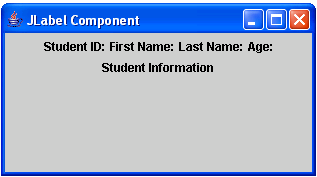
Here's the full code of this tutorial:
- import
java.awt.*
;
// used to access the FlowLayout Class
- import
javax.swing.*
;
//used to access the JFrame and JLabel class
- public
class
jLabelComponent {
- public
static
void
main(
String
[
]
args)
{
- JFrame
frame =
new
JFrame
(
"JLabel Component"
)
;
- JLabel
label1 =
new
JLabel
(
"Student ID:"
, JLabel
.LEFT
)
;
- JLabel
label2 =
new
JLabel
(
"First Name:"
, JLabel
.RIGHT
)
;
- JLabel
label3 =
new
JLabel
(
"Last Name:"
, JLabel
.RIGHT
)
;
- JLabel
label4 =
new
JLabel
(
"Age:"
, JLabel
.LEFT
)
;
- JLabel
label5 =
new
JLabel
(
"Student Information"
, JLabel
.CENTER
)
;
- label5.setVerticalAlignment
(
JLabel
.CENTER
)
;
- label5.setToolTipText
(
"This is a label!"
)
;
- frame.getContentPane
(
)
.add
(
label1)
;
- frame.getContentPane
(
)
.add
(
label2)
;
- frame.getContentPane
(
)
.add
(
label3)
;
- frame.getContentPane
(
)
.add
(
label4)
;
- frame.getContentPane
(
)
.add
(
label5)
;
- frame.setSize
(
300
, 300
)
;
- frame.setVisible
(
true
)
;
- frame.setDefaultCloseOperation
(
JFrame
.EXIT_ON_CLOSE
)
;
- frame.getContentPane
(
)
.setLayout
(
new
FlowLayout
(
)
)
;
- }
- }
For more inquiries and need programmer for your thesis systems in any kind of programming languages, just contact my number below.
Best Regards,
Engr. Lyndon Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
You must upgrade your account or reply in the thread to view the hidden content.