Lucifer636
Execution Flow Analyzer
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
100 XP
In this tutorial, i will going to teach you how to create a program that computes factorial of a number. We all know that factorial is defined only for natural numbers and it means as the continued product of successive natural numbers from 1 to the number inputted.
Now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of factorial.java.
2. Import javax.swing package. Hence we will use a GUI (Graphical User Interface) here like the inputting the number.
3. Initialize your variable in your Main, variable numInput as string and make it as an input and variable n, i, f as int. n variable will be used for parsing the numInput variable to be int, i for the loop, and variable f for the factorial of a number.
4. Make a for loop statement that the parameters have variable i will be equal to variable n, i is greater or equal than 1, and will decrement. Also, make f is equal to 1, so that we will have the formula of f=f*1 to get the factorial.
5. Lastly, display the output of the program using JOptionPane.showMessageDialog.
Output:
Solution:
1 x 2 x 3 x 4 x 5 = 120
Here's the full code of this tutorial:
Hope this helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
Now let's start this tutorial!
1. Open JCreator or NetBeans and make a java program with a file name of factorial.java.
2. Import javax.swing package. Hence we will use a GUI (Graphical User Interface) here like the inputting the number.
- import
javax.swing.*
;
3. Initialize your variable in your Main, variable numInput as string and make it as an input and variable n, i, f as int. n variable will be used for parsing the numInput variable to be int, i for the loop, and variable f for the factorial of a number.
- int
n,i,f;
- String
numInput;
- numInput =
JOptionPane
.showInputDialog
(
null
, "Enter a number:"
)
;
- n =
Integer
.parseInt
(
numInput)
;
4. Make a for loop statement that the parameters have variable i will be equal to variable n, i is greater or equal than 1, and will decrement. Also, make f is equal to 1, so that we will have the formula of f=f*1 to get the factorial.
- f =
1
;
- for
(
i=
n;
i>=
1
;
i--
)
{
- f =
f*
i;
- }
5. Lastly, display the output of the program using JOptionPane.showMessageDialog.
- JOptionPane
.showMessageDialog
(
null
, "Factorial value is: "
+
f)
;
- }
Output:
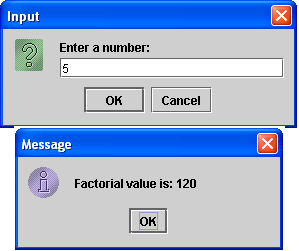
Solution:
1 x 2 x 3 x 4 x 5 = 120
Here's the full code of this tutorial:
- import
javax.swing.*
;
- public
class
factorial {
- public
static
void
main(
String
[
]
args)
{
- int
n,i,f;
- String
numInput;
- numInput =
JOptionPane
.showInputDialog
(
null
, "Enter a number:"
)
;
- n =
Integer
.parseInt
(
numInput)
;
- //... Computation
- f =
1
;
- for
(
i=
n;
i>=
1
;
i--
)
{
- f =
f*
i;
- }
- //... Output
- JOptionPane
.showMessageDialog
(
null
, "Factorial value is: "
+
f)
;
- }
- }
Hope this helps! :)
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Download
You must upgrade your account or reply in the thread to view the hidden content.