Ashwathama
OpenAPI Spec Writer
LEVEL 1
200 XP
This is my final continuation of my other two tutorials: Creating User Account Information in Java - Adding Records to Database and Creating User Account Information in Java - Searching Records to Database. Now, we don't have to modify our code for that. Only add the following code below to your UserSettings.java.
1. This is for the initialization of variables to be used as buttons and labels. Just use JButton to have a button in your form. Here we just add Update, Delete, and Exit Button from the previous tutorial.
2. Now in your constructor UserSettings() , add the 3 buttons for update, delete, and exit.
3. Lastly, in the actionEvents when clicking this 3 buttons put this code below.
The syntax for updating a record here is the: PreparedStatement
ps =
cn.prepareStatement
(
"UPDATE Login SET password = '"
+
txtPass.getText
(
)
+
"',name1 = '"
+
txtName1.getText
(
)
+
"',name2= '"
+
txtName2.getText
(
)
+
"'WHERE username = '"
+
txtUser.getText
(
)
+
"'"
)
;
The syntax for deleting a record here is the: PreparedStatement
ps =
cn.prepareStatement
(
"DELETE FROM Login WHERE username ='"
+
txtUser.getText
(
)
+
"'"
)
;
And lastly, the dispose() function is used for exiting or closing the form.
Output:
Here is the full code of this tutorial:
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Download
1. This is for the initialization of variables to be used as buttons and labels. Just use JButton to have a button in your form. Here we just add Update, Delete, and Exit Button from the previous tutorial.
- public
class
UserSettings extends
JFrame
implements
ActionListener
{
- JButton
btnNew =
new
JButton
(
"Add"
)
;
- JButton
btnUpdate =
new
JButton
(
"Update"
)
;
- JButton
btnDelete =
new
JButton
(
"Delete"
)
;
- JButton
btnSearch =
new
JButton
(
"Search"
)
;
- JButton
btnExit =
new
JButton
(
"Exit"
)
;
- }
2. Now in your constructor UserSettings() , add the 3 buttons for update, delete, and exit.
- public
UserSettings(
)
{
- btnUpdate.setBounds
(
80
,190
,75
,35
)
;
- pane.add
(
btnUpdate)
;
- btnUpdate.addActionListener
(
this
)
;
- btnDelete.setBounds
(
155
,190
,75
,35
)
;
- pane.add
(
btnDelete)
;
- btnDelete.addActionListener
(
this
)
;
- btnExit.setBounds
(
130
,260
,75
,35
)
;
- pane.add
(
btnExit)
;
- }
3. Lastly, in the actionEvents when clicking this 3 buttons put this code below.
- if
(
source ==
btnUpdate)
{
- try
{
- String
uname=
txtUser.getText
(
)
;
- String
pass=
txtPass.getText
(
)
;
- String
name1=
txtName1.getText
(
)
;
- String
name2=
txtName2.getText
(
)
;
- if
(
!
uname.equals
(
""
)
&&
!
pass.equals
(
""
)
&&
!
name1.equals
(
""
)
&&
!
name2.equals
(
""
)
)
{
- st=
cn.createStatement
(
)
;
- PreparedStatement
ps =
cn.prepareStatement
(
"UPDATE Login SET password = '"
+
txtPass.getText
(
)
+
"',name1 = '"
+
txtName1.getText
(
)
+
"',name2= '"
+
txtName2.getText
(
)
+
"'WHERE username = '"
+
txtUser.getText
(
)
+
"'"
)
;
- ps.executeUpdate
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"Account has been successfully updated."
,"Payroll System: User settings"
,JOptionPane
.INFORMATION_MESSAGE
)
;
- txtUser.requestFocus
(
true
)
;
- clear(
)
;
- st.close
(
)
;
- }
- else
{
- JOptionPane
.showMessageDialog
(
null
,"Please Fill Up The Empty Fields"
,"Warning"
,JOptionPane
.WARNING_MESSAGE
)
;
- }
- }
catch
(
SQLException
y)
{
- JOptionPane
.showMessageDialog
(
null
,"Unable to update!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
- }
- }
- if
(
source==
btnDelete)
{
- try
{
- PreparedStatement
ps =
cn.prepareStatement
(
"DELETE FROM Login WHERE username ='"
+
txtUser.getText
(
)
+
"'"
)
;
- ps.executeUpdate
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"Account has been successfully deleted."
,"Payroll System: User settings "
,JOptionPane
.INFORMATION_MESSAGE
)
;
- txtUser.requestFocus
(
true
)
;
- clear(
)
;
- st.close
(
)
;
- }
catch
(
SQLException
s)
{
- JOptionPane
.showMessageDialog
(
null
,"Unable to delete!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
}
- }
if
(
source==
btnExit)
{
- dispose(
)
;
- }
- }
The syntax for updating a record here is the: PreparedStatement
ps =
cn.prepareStatement
(
"UPDATE Login SET password = '"
+
txtPass.getText
(
)
+
"',name1 = '"
+
txtName1.getText
(
)
+
"',name2= '"
+
txtName2.getText
(
)
+
"'WHERE username = '"
+
txtUser.getText
(
)
+
"'"
)
;
The syntax for deleting a record here is the: PreparedStatement
ps =
cn.prepareStatement
(
"DELETE FROM Login WHERE username ='"
+
txtUser.getText
(
)
+
"'"
)
;
And lastly, the dispose() function is used for exiting or closing the form.
Output:
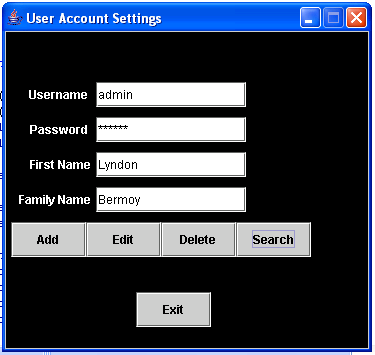
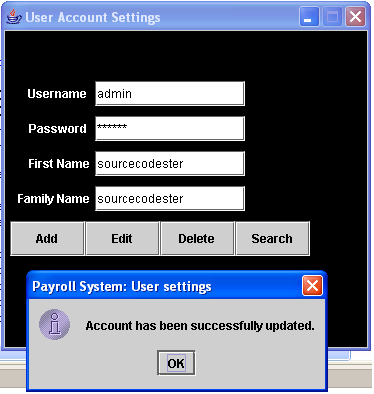
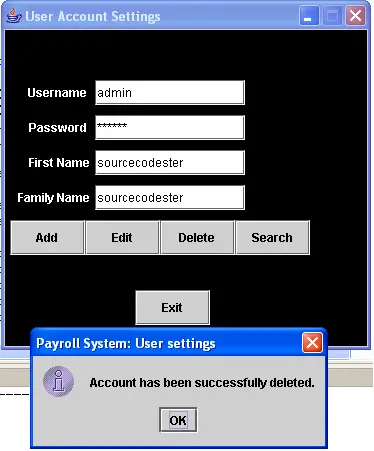
Here is the full code of this tutorial:
- import
javax.swing.*
;
- import
java.awt.*
;
- import
java.awt.event.*
;
- import
java.sql.*
;
- import
java.lang.*
;
- public
class
UserSettings extends
JFrame
implements
ActionListener
{
- JLabel
lblUser =
new
JLabel
(
"Username "
,JLabel
.RIGHT
)
;
- JLabel
lblPass =
new
JLabel
(
"Password "
,JLabel
.RIGHT
)
;
- JLabel
lblName1 =
new
JLabel
(
"First Name"
,JLabel
.RIGHT
)
;
- JLabel
lblName2 =
new
JLabel
(
"Family Name"
,JLabel
.RIGHT
)
;
- JTextField
txtUser =
new
JTextField
(
20
)
;
- JPasswordField
txtPass=
new
JPasswordField
(
20
)
;
lll
- JTextField
txtName1=
new
JTextField
(
20
)
;
- JTextField
txtName2=
new
JTextField
(
20
)
;
- JButton
btnNew =
new
JButton
(
"Add"
)
;
- JButton
btnUpdate =
new
JButton
(
"Edit"
)
;
- JButton
btnDelete =
new
JButton
(
"Delete"
)
;
- JButton
btnSearch =
new
JButton
(
"Search"
)
;
- JButton
btnExit =
new
JButton
(
"Exit"
)
;
- Connection
cn;
- Statement
st;
- PreparedStatement
ps;
- public
void
clear(
)
{
- txtUser.setText
(
""
)
;
- txtPass.setText
(
""
)
;
- txtName1.setText
(
""
)
;
- txtName2.setText
(
""
)
;
- }
- public
UserSettings(
)
{
- super
(
"User Account Settings"
)
;
- JPanel
pane =
new
JPanel
(
)
;
- pane.setLayout
(
null
)
;
- lblUser.setBounds
(
5
,50
,80
,25
)
;
- pane.add
(
lblUser)
;
- txtUser.setBounds
(
90
,50
,150
,25
)
;
- pane.add
(
txtUser)
;
- lblUser.setForeground
(
Color
.white
)
;
- lblPass.setBounds
(
5
,85
,80
,25
)
;
- pane.add
(
lblPass)
;
- txtPass.setBounds
(
90
,85
,150
,25
)
;
- txtPass.setEchoChar
(
'*'
)
;
- pane.add
(
txtPass)
;
- lblPass.setForeground
(
Color
.white
)
;
- lblName1.setBounds
(
5
,120
,80
,25
)
;
- pane.add
(
lblName1)
;
- txtName1.setBounds
(
90
,120
,150
,25
)
;
- pane.add
(
txtName1)
;
- lblName1.setForeground
(
Color
.white
)
;
- lblName2.setBounds
(
5
,155
,80
,25
)
;
- pane.add
(
lblName2)
;
- txtName2.setBounds
(
90
,155
,150
,25
)
;
- pane.add
(
txtName2)
;
- lblName2.setForeground
(
Color
.white
)
;
- btnNew.setBounds
(
5
,190
,75
,35
)
;
- pane.add
(
btnNew)
;
- btnNew.addActionListener
(
this
)
;
- btnUpdate.setBounds
(
80
,190
,75
,35
)
;
- pane.add
(
btnUpdate)
;
- btnUpdate.addActionListener
(
this
)
;
- btnDelete.setBounds
(
155
,190
,75
,35
)
;
- pane.add
(
btnDelete)
;
- btnDelete.addActionListener
(
this
)
;
- btnSearch.setBounds
(
230
,190
,75
,35
)
;
- pane.add
(
btnSearch)
;
- btnSearch.addActionListener
(
this
)
;
- btnExit.setBounds
(
130
,260
,75
,35
)
;
- pane.add
(
btnExit)
;
- pane.setBackground
(
Color
.black
)
;
- btnExit.addActionListener
(
this
)
;
- setContentPane(
pane)
;
- setDefaultCloseOperation(
JFrame
.DISPOSE_ON_CLOSE
)
;
- pane.setBorder
(
BorderFactory
.createTitledBorder
(
- BorderFactory
.createEtchedBorder
(
)
, "Creating User Account"
)
)
;
- try
{
- Class
.forName
(
"sun.jdbc.odbc.JdbcOdbcDriver"
)
;
- cn =
DriverManager
.getConnection
(
"jdbc:odbc:User"
)
;
- }
catch
(
ClassNotFoundException
e)
{
- System
.err
.println
(
"Failed to load driver"
)
;
- e.printStackTrace
(
)
;
- }
- catch
(
SQLException
e)
{
- System
.err
.println
(
"Unable to connect"
)
;
- e.printStackTrace
(
)
;
- }
- }
- public
void
actionPerformed(
ActionEvent
e)
{
- Object
source =
e.getSource
(
)
;
- if
(
source ==
btnNew)
{
- try
{
- String
uname=
txtUser.getText
(
)
;
- String
pass=
txtPass.getText
(
)
;
- String
name1=
txtName1.getText
(
)
;
- String
name2=
txtName2.getText
(
)
;
- if
(
!
uname.equals
(
""
)
&&
!
pass.equals
(
""
)
&&
!
name1.equals
(
""
)
&&
!
name2.equals
(
""
)
)
{
- st=
cn.createStatement
(
)
;
- ps=
cn.prepareStatement
(
"INSERT INTO Login"
+
" (username,password,name1,name2) "
+
" VALUES(?,?,?,?)"
)
;
- ps.setString
(
1
,txtUser.getText
(
)
)
;
- ps.setString
(
2
,txtPass.getText
(
)
)
;
- ps.setString
(
3
,txtName1.getText
(
)
)
;
- ps.setString
(
4
,txtName2.getText
(
)
)
;
- ps.executeUpdate
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"New account has been successfully added."
,"Payroll System: User settings"
,JOptionPane
.INFORMATION_MESSAGE
)
;
- txtUser.requestFocus
(
true
)
;
- st.close
(
)
;
- clear(
)
;
- }
- else
{
- JOptionPane
.showMessageDialog
(
null
,"Please Fill Up The Empty Fields"
,"Warning"
,JOptionPane
.WARNING_MESSAGE
)
;
- }
- }
catch
(
SQLException
sqlEx)
{
- sqlEx.printStackTrace
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"Unable to save!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
}
- }
- if
(
source ==
btnSearch)
{
- try
{
- String
sUser =
""
;
- int
tmp=
0
;
- clear(
)
;
- sUser =
JOptionPane
.showInputDialog
(
null
,"Enter Username to search."
,"Payroll System: User settings"
,JOptionPane
.QUESTION_MESSAGE
)
;
- st=
cn.createStatement
(
)
;
- ResultSet
rs=
st.executeQuery
(
"SELECT * FROM Login WHERE username = '"
+
sUser +
"'"
)
;
- while
(
rs.next
(
)
)
{
- txtUser.setText
(
rs.getString
(
1
)
)
;
- txtPass.setText
(
rs.getString
(
2
)
)
;
- txtName1.setText
(
rs.getString
(
3
)
)
;
- txtName2.setText
(
rs.getString
(
4
)
)
;
- tmp=
1
;
- }
- st.close
(
)
;
- if
(
tmp==
0
)
{
- JOptionPane
.showMessageDialog
(
null
,"No record found!!."
,"Payroll System: User settings"
,JOptionPane
.INFORMATION_MESSAGE
)
;
- }
- }
catch
(
SQLException
s)
{
- JOptionPane
.showMessageDialog
(
null
,"Unable to search!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
- System
.out
.println
(
"SQL Error"
+
s.toString
(
)
+
" "
+
s.getErrorCode
(
)
+
" "
+
s.getSQLState
(
)
)
;
- }
- }
- if
(
source ==
btnUpdate)
{
- try
{
- String
uname=
txtUser.getText
(
)
;
- String
pass=
txtPass.getText
(
)
;
- String
name1=
txtName1.getText
(
)
;
- String
name2=
txtName2.getText
(
)
;
- if
(
!
uname.equals
(
""
)
&&
!
pass.equals
(
""
)
&&
!
name1.equals
(
""
)
&&
!
name2.equals
(
""
)
)
{
- st=
cn.createStatement
(
)
;
- PreparedStatement
ps =
cn.prepareStatement
(
"UPDATE Login SET password = '"
+
txtPass.getText
(
)
+
"',name1 = '"
+
txtName1.getText
(
)
+
"',name2= '"
+
txtName2.getText
(
)
+
"'WHERE username = '"
+
txtUser.getText
(
)
+
"'"
)
;
- ps.executeUpdate
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"Account has been successfully updated."
,"Payroll System: User settings"
,JOptionPane
.INFORMATION_MESSAGE
)
;
- txtUser.requestFocus
(
true
)
;
- clear(
)
;
- st.close
(
)
;
- }
- else
{
- JOptionPane
.showMessageDialog
(
null
,"Please Fill Up The Empty Fields"
,"Warning"
,JOptionPane
.WARNING_MESSAGE
)
;
- }
- }
catch
(
SQLException
y)
{
- JOptionPane
.showMessageDialog
(
null
,"Unable to update!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
- }
- }
- if
(
source==
btnDelete)
{
- try
{
- PreparedStatement
ps =
cn.prepareStatement
(
"DELETE FROM Login WHERE username ='"
+
txtUser.getText
(
)
+
"'"
)
;
- ps.executeUpdate
(
)
;
- JOptionPane
.showMessageDialog
(
null
,"Account has been successfully deleted."
,"Payroll System: User settings "
,JOptionPane
.INFORMATION_MESSAGE
)
;
- txtUser.requestFocus
(
true
)
;
- clear(
)
;
- st.close
(
)
;
- }
catch
(
SQLException
s)
{
- JOptionPane
.showMessageDialog
(
null
,"Unable to delete!."
,"Payroll System: User settings"
,JOptionPane
.ERROR_MESSAGE
)
;
}
- }
if
(
source==
btnExit)
{
- dispose(
)
;
- }
- }
- // public void frameUser(){
- public
static
void
main(
String
[
]
args)
{
- UserSettings panel =
new
UserSettings(
)
;
- panel.setSize
(
370
,350
)
;
- panel.setVisible
(
true
)
;
- panel.setLocation
(
350
,200
)
;
- panel.setResizable
(
false
)
;
- }
- }
Best Regards,
Engr. Lyndon R. Bermoy
IT Instructor/System Developer/Android Developer/Freelance Programmer
If you have some queries, feel free to contact the number or e-mail below.
Mobile: 09488225971
Landline: 826-9296
E-mail:[email protected]
Visit and like my page on Facebook at: https://www.facebook.com/BermzISware
Add and Follow me on Facebook: https://www.facebook.com/donzzsky
Download
You must upgrade your account or reply in the thread to view the hidden content.