0hSally
Grey Hat
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
300 XP
This time, I will teach you how to navigate records based on ListView in C# and MySQL Database. This method has the ability to navigate records in the ListView. It also provides a next and previous button that allows the movement of records back and forth. In this way, you can control the data to be displayed in the listview.
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.
Step 2
Do the form just like shown below.
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL
libraries
Step 4
Establish a connection between C# and MySQL database. After that, declare all the classes and variables that are needed.
Step 5
Create a method for retrieving single data in the database.
Step 6
Create a method for retrieving list of data in the database
Step 7
Create a method for the next records.
Step 8
Create a method for the previous records
Step 9
Write the following codes for retrieving and displaying data in the first load of the form.
Step 10
Write the following codes for the next button
Step 11
Write the following codes for the previous button
The complete source code is included you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Download
Creating Application
Step 1
Open Microsoft Visual Studio 2015 and create a new windows form application for c#.
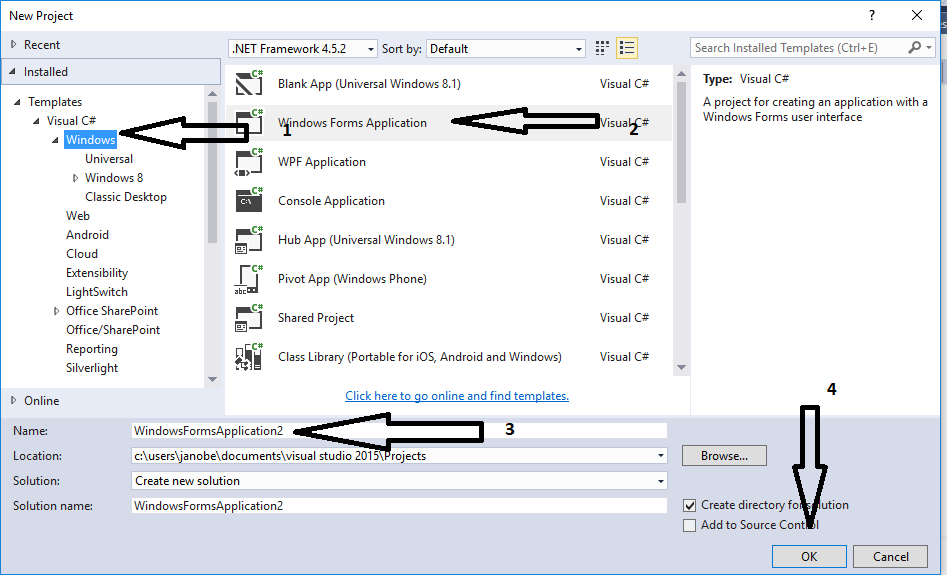
Step 2
Do the form just like shown below.
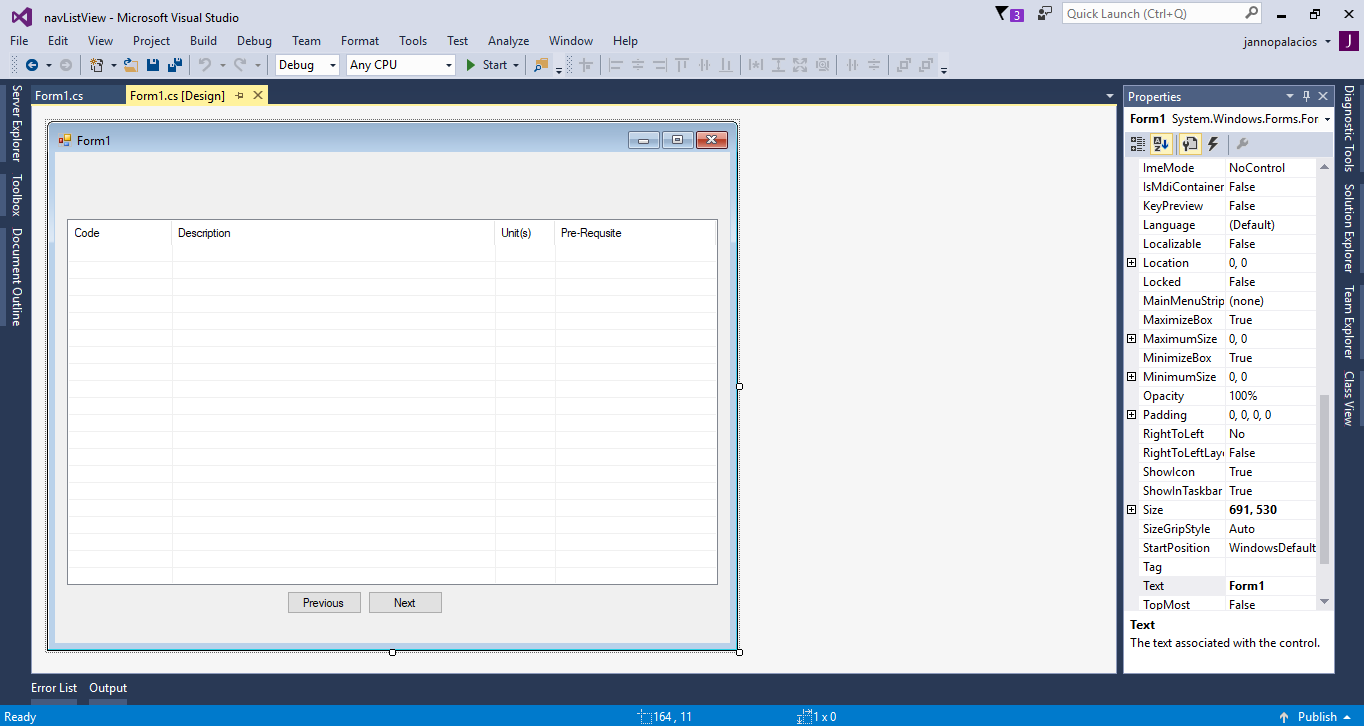
Step 3
Press F7 to open the code editor. In the code editor, add a namespace to access MySQL
libraries
- using
MySql.Data.MySqlClient
;
Step 4
Establish a connection between C# and MySQL database. After that, declare all the classes and variables that are needed.
- MySqlConnection con =
new
MySqlConnection(
"server=localhost;user id=root;password=;database=dbsubjects;sslMode=none"
)
;
- MySqlCommand cmd;
- MySqlDataAdapter da;
- DataTable dt;
- String
sql;
- int
maxrow;
- int
inc_value;
- int
resultPerPage =
10
;
- int
startResult;
Step 5
Create a method for retrieving single data in the database.
- private
void
loadSingleResult(
String
sql)
- {
- try
- {
- con.
Open
(
)
;
- cmd =
new
MySqlCommand(
)
;
- da =
new
MySqlDataAdapter(
)
;
- dt =
new
DataTable(
)
;
- cmd.
Connection
=
con;
- cmd.
CommandText
=
sql;
- da.
SelectCommand
=
cmd;
- da.
Fill
(
dt)
;
- maxrow =
dt.
Rows
.
Count
-
1
;
- }
- catch
(
Exception ex)
- {
- MessageBox.
Show
(
ex.
Message
)
;
- }
- finally
- {
- con.
Close
(
)
;
- da.
Dispose
(
)
;
- }
- }
Step 6
Create a method for retrieving list of data in the database
- private
void
loadResultList(
String
sql, ListView lst)
- {
- try
- {
- con.
Open
(
)
;
- cmd =
new
MySqlCommand(
)
;
- da =
new
MySqlDataAdapter(
)
;
- dt =
new
DataTable(
)
;
- cmd.
Connection
=
con;
- cmd.
CommandText
=
sql;
- da.
SelectCommand
=
cmd;
- da.
Fill
(
dt)
;
- lst.
Items
.
Clear
(
)
;
- foreach
(
DataRow r in
dt.
Rows
)
- {
- var
list =
lst.
Items
.
Add
(
r.
Field
<
string
>
(
1
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
string
>
(
2
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
int
>
(
3
)
.
ToString
(
)
)
;
- list.
SubItems
.
Add
(
r.
Field
<
string
>
(
4
)
.
ToString
(
)
)
;
- }
- }
- catch
(
Exception ex)
- {
- MessageBox.
Show
(
ex.
Message
)
;
- }
- finally
- {
- con.
Close
(
)
;
- da.
Dispose
(
)
;
- }
- }
Step 7
Create a method for the next records.
- private
void
next_record(
)
- {
- if
(
inc_value !=
maxrow -
2
)
- {
- inc_value =
inc_value +
1
;
- startResult =
inc_value *
resultPerPage;
- }
- else
- {
- if
(
inc_value ==
maxrow)
- {
- return
;
- }
- }
- sql =
"Select * from subject limit "
+
startResult +
","
+
resultPerPage +
""
;
- loadResultList(
sql, listView1)
;
- }
Step 8
Create a method for the previous records
- private
void
previous_record(
)
- {
- if
(
inc_value ==
0
)
- {
- return
;
- }
- else
if
(
inc_value >
0
)
- {
- inc_value =
inc_value -
1
;
- startResult =
inc_value *
resultPerPage;
- }
- sql =
"Select * from subject limit "
+
startResult +
","
+
resultPerPage +
""
;
- loadResultList(
sql, listView1)
;
- }
Step 9
Write the following codes for retrieving and displaying data in the first load of the form.
- private
void
Form1_Load(
object
sender, EventArgs e)
- {
- sql =
"Select * from subject"
;
- loadSingleResult(
sql)
;
- sql =
"Select * from subject limit 10"
;
- loadResultList(
sql, listView1)
;
- }
Step 10
Write the following codes for the next button
- private
void
btn_next_Click(
object
sender, EventArgs e)
- {
- next_record(
)
;
- }
Step 11
Write the following codes for the previous button
- private
void
btn_previous_Click(
object
sender, EventArgs e)
- {
- previous_record(
)
;
- }
The complete source code is included you can download it and run it on your computer.
For any questions about this article. You can contact me @
Email – [email protected]
Mobile No. – 09305235027 – TNT
Or feel free to comment below.
Download
You must upgrade your account or reply in the thread to view hidden text.