bonestherapper
Product Launch Expert
2
MONTHS
2 2 MONTHS OF SERVICE
LEVEL 1
200 XP
In this tutorial we will create a Simple Digital Clock using C#. C# is a general-purpose, object-oriented programming language. C# automatically manages inaccessible object memory using a garbage collector, which eliminates developer concerns and memory leaks. It has a designed for improving productivity in the development of Web applications. It has a friendly environment for all new developers. So let's do the coding...
Getting Started
First you will have to download & install the Visual Studio. Visual Studios is an open source development feel free to create any application that you want.
Here's the link for the Visual Studio https://www.visualstudio.com/.
Application Design
We will now create the design for the application, first locate the designer file called form1.Designer.cs, this is the default name when you create a new windows form. Rename the form as Main.cs and then write these codes inside your designer file.
Creating the Script
We will now create the script to make things work. To do first add a timer tools in the form, then go to the timer properties and set enabled to true.
After that go to the csharp script called Main.cs then right click and select view code, this will force you to go to the text editor. Then write these block of codes inside the Class of the form.
Try to run the application and see if it works.
There you go we successfully created a Simple Digital Clock using C#. I hope that this tutorial help you understand on how to develop an application using C#. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Download
Getting Started
First you will have to download & install the Visual Studio. Visual Studios is an open source development feel free to create any application that you want.
Here's the link for the Visual Studio https://www.visualstudio.com/.
Application Design
We will now create the design for the application, first locate the designer file called form1.Designer.cs, this is the default name when you create a new windows form. Rename the form as Main.cs and then write these codes inside your designer file.
- namespace
Simple_Digital_Clock
- {
- partial
class
Main
- {
- /// <summary>
- /// Required designer variable.
- /// </summary>
- private
System.ComponentModel
.
IContainer
components =
null
;
- /// <summary>
- /// Clean up any resources being used.
- /// </summary>
- /// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
- protected
override
void
Dispose(
bool
disposing)
- {
- if
(
disposing &&
(
components !=
null
)
)
- {
- components.
Dispose
(
)
;
- }
- base
.
Dispose
(
disposing)
;
- }
- #region Windows Form Designer generated code
- /// <summary>
- /// Required method for Designer support - do not modify
- /// the contents of this method with the code editor.
- /// </summary>
- private
void
InitializeComponent(
)
- {
- this
.
components
=
new
System.ComponentModel
.
Container
(
)
;
- this
.
lbl_time
=
new
System
.
Windows
.
Forms
.
Label
(
)
;
- this
.
lbl_sec
=
new
System
.
Windows
.
Forms
.
Label
(
)
;
- this
.
lbl_day
=
new
System
.
Windows
.
Forms
.
Label
(
)
;
- this
.
timer1
=
new
System
.
Windows
.
Forms
.
Timer
(
this
.
components
)
;
- this
.
lbl_date
=
new
System
.
Windows
.
Forms
.
Label
(
)
;
- this
.
SuspendLayout
(
)
;
- //
- // lbl_time
- //
- this
.
lbl_time
.
AutoSize
=
true
;
- this
.
lbl_time
.
Font
=
new
System.Drawing
.
Font
(
"DS-Digital"
, 72F, System.Drawing
.
FontStyle
.
Regular
, System.Drawing
.
GraphicsUnit
.
Point
, (
(
byte
)
(
0
)
)
)
;
- this
.
lbl_time
.
ForeColor
=
System.Drawing
.
Color
.
Green
;
- this
.
lbl_time
.
Location
=
new
System.Drawing
.
Point
(
88
, 76
)
;
- this
.
lbl_time
.
Name
=
"lbl_time"
;
- this
.
lbl_time
.
Size
=
new
System.Drawing
.
Size
(
376
, 142
)
;
- this
.
lbl_time
.
TabIndex
=
0
;
- this
.
lbl_time
.
Text
=
"00:00"
;
- //
- // lbl_sec
- //
- this
.
lbl_sec
.
AutoSize
=
true
;
- this
.
lbl_sec
.
Font
=
new
System.Drawing
.
Font
(
"DS-Digital"
, 20F, System.Drawing
.
FontStyle
.
Regular
, System.Drawing
.
GraphicsUnit
.
Point
, (
(
byte
)
(
0
)
)
)
;
- this
.
lbl_sec
.
ForeColor
=
System.Drawing
.
Color
.
Green
;
- this
.
lbl_sec
.
Location
=
new
System.Drawing
.
Point
(
423
, 161
)
;
- this
.
lbl_sec
.
Name
=
"lbl_sec"
;
- this
.
lbl_sec
.
Size
=
new
System.Drawing
.
Size
(
57
, 40
)
;
- this
.
lbl_sec
.
TabIndex
=
1
;
- this
.
lbl_sec
.
Text
=
"00"
;
- //
- // lbl_day
- //
- this
.
lbl_day
.
AutoSize
=
true
;
- this
.
lbl_day
.
Font
=
new
System.Drawing
.
Font
(
"DS-Digital"
, 20F, System.Drawing
.
FontStyle
.
Regular
, System.Drawing
.
GraphicsUnit
.
Point
, (
(
byte
)
(
0
)
)
)
;
- this
.
lbl_day
.
ForeColor
=
System.Drawing
.
Color
.
Green
;
- this
.
lbl_day
.
Location
=
new
System.Drawing
.
Point
(
343
, 201
)
;
- this
.
lbl_day
.
Name
=
"lbl_day"
;
- this
.
lbl_day
.
Size
=
new
System.Drawing
.
Size
(
77
, 40
)
;
- this
.
lbl_day
.
TabIndex
=
2
;
- this
.
lbl_day
.
Text
=
"Day"
;
- //
- // timer1
- //
- this
.
timer1
.
Enabled
=
true
;
- this
.
timer1
.
Tick
+=
new
System
.
EventHandler
(
this
.
Time_Start
)
;
- //
- // lbl_date
- //
- this
.
lbl_date
.
AutoSize
=
true
;
- this
.
lbl_date
.
Font
=
new
System.Drawing
.
Font
(
"DS-Digital"
, 20F, System.Drawing
.
FontStyle
.
Regular
, System.Drawing
.
GraphicsUnit
.
Point
, (
(
byte
)
(
0
)
)
)
;
- this
.
lbl_date
.
ForeColor
=
System.Drawing
.
Color
.
Green
;
- this
.
lbl_date
.
Location
=
new
System.Drawing
.
Point
(
105
, 200
)
;
- this
.
lbl_date
.
Name
=
"lbl_date"
;
- this
.
lbl_date
.
Size
=
new
System.Drawing
.
Size
(
171
, 40
)
;
- this
.
lbl_date
.
TabIndex
=
3
;
- this
.
lbl_date
.
Text
=
"MM-DD-YY"
;
- //
- // Main
- //
- this
.
AutoScaleDimensions
=
new
System.Drawing
.
SizeF
(
9F, 20F)
;
- this
.
AutoScaleMode
=
System
.
Windows
.
Forms
.
AutoScaleMode
.
Font
;
- this
.
BackColor
=
System.Drawing
.
Color
.
Black
;
- this
.
ClientSize
=
new
System.Drawing
.
Size
(
557
, 342
)
;
- this
.
Controls
.
Add
(
this
.
lbl_date
)
;
- this
.
Controls
.
Add
(
this
.
lbl_day
)
;
- this
.
Controls
.
Add
(
this
.
lbl_sec
)
;
- this
.
Controls
.
Add
(
this
.
lbl_time
)
;
- this
.
ForeColor
=
System.Drawing
.
SystemColors
.
ActiveCaptionText
;
- this
.
FormBorderStyle
=
System
.
Windows
.
Forms
.
FormBorderStyle
.
None
;
- this
.
Name
=
"Main"
;
- this
.
StartPosition
=
System
.
Windows
.
Forms
.
FormStartPosition
.
CenterScreen
;
- this
.
Text
=
"Form1"
;
- this
.
ResumeLayout
(
false
)
;
- this
.
PerformLayout
(
)
;
- }
- #endregion
- private
System
.
Windows
.
Forms
.
Label
lbl_time;
- private
System
.
Windows
.
Forms
.
Label
lbl_sec;
- private
System
.
Windows
.
Forms
.
Label
lbl_day;
- private
System
.
Windows
.
Forms
.
Timer
timer1;
- private
System
.
Windows
.
Forms
.
Label
lbl_date;
- }
- }
Creating the Script
We will now create the script to make things work. To do first add a timer tools in the form, then go to the timer properties and set enabled to true.
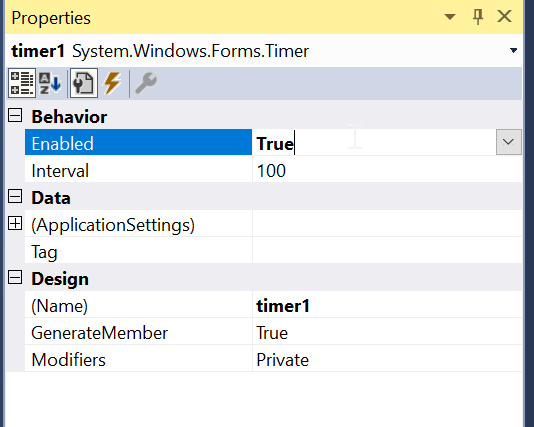
After that go to the csharp script called Main.cs then right click and select view code, this will force you to go to the text editor. Then write these block of codes inside the Class of the form.
- using
System
;
- using
System.Collections.Generic
;
- using
System.ComponentModel
;
- using
System.Data
;
- using
System.Drawing
;
- using
System.Linq
;
- using
System.Text
;
- using
System.Threading.Tasks
;
- using
System.Windows.Forms
;
- namespace
Simple_Digital_Clock
- {
- public
partial
class
Main :
Form
- {
- public
Main(
)
- {
- InitializeComponent(
)
;
- }
- private
void
Time_Start(
object
sender, EventArgs e)
- {
- lbl_time.
Text
=
DateTime.
Now
.
ToString
(
"hh:mm"
)
;
- lbl_sec.
Text
=
DateTime.
Now
.
ToString
(
"ss"
)
;
- lbl_day.
Text
=
DateTime.
Now
.
ToString
(
"dddd"
)
;
- lbl_date.
Text
=
DateTime.
Now
.
ToString
(
"MMM dd yyyy"
)
;
- }
- }
- }
Try to run the application and see if it works.
There you go we successfully created a Simple Digital Clock using C#. I hope that this tutorial help you understand on how to develop an application using C#. For more updates and tutorials just kindly visit this site. Enjoy Coding!!!
Download
You must upgrade your account or reply in the thread to view hidden text.